The following article describes the Difference Between continue and break Statements in Java.
In Java, the continue
and break
statements are used to control the flow of a loop.
When we need to skip the current iteration, we use the continue
statement. As a result of executing the continue statement, the loop moves to the next iteration.For example, if you have a loop that is iterating over an array, and you want to skip the current iteration if a certain condition is met, you can use a continue
statement.
The break
statement, on the other hand, is used to exit the loop completely. Once a break
statement is executed, the loop terminates and the program continues executing the code that follows the loop. Here is an example of using continue
and break
in a for loop:
for (int i = 20; i < 100; i+=10) {
if (i == 50) {
continue;
}
System.out.println(i);
if (i == 70) {
break;
}
}
The output of this code will be:
20
30
40
60
When i becomes 50, the loop doesn’t print it. Bcause the continue statement skips that iteration. Similarly, when i becomes 70, the loop terminates. This is because of break statement.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
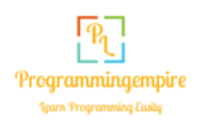