The following article describes what is a data class in Kotlin, and how is it used.
In Kotlin, a data class is a special type of class that is designed to hold data. It is commonly used to represent data structures such as records, DTOs (data transfer objects), or model classes.
Data classes in Kotlin are similar to Java’s POJO (Plain Old Java Object) or Java’s JavaBeans, but they provide a more concise and declarative way to define data structures with built-in support for some useful features, such as:
- Automatic generation of toString(), equals(), hashCode(), and copy() methods: Data classes automatically generate these methods based on the properties defined in the class.
- Property accessors: Data classes automatically generate getters and setters for properties defined in the class.
- Destructuring declarations: Data classes allow you to easily destructure an instance into its component parts, making it easy to extract and use the data held in the class.
Here’s an example of a data class in Kotlin:
data class User(val id: Int, val name: String, val email: String)
In this example, we define a data class called User
with three properties: id
, name
, and email
. The data
keyword before the class declaration tells the compiler to generate the methods for toString(), equals(), hashCode(), and copy() based on the properties defined in the class.
With this simple declaration, you can create instances of the User
class, compare them for equality, use them in collections and other data structures, and destructure them to extract their properties, among other things.
Data classes in Kotlin provide a concise and powerful way to define data structures, making it easier to write and maintain code that deals with complex data.
Further Reading
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Data Science
- Data Structures and Algorithms
- Deep Learning
- Django
- Dot Net Framework
- Drones
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Smart City
- Software
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
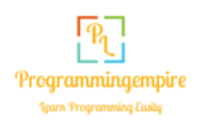