The following article describes Creating Matrix in PHP.
In PHP, you can create a matrix using a 2D array. The following example shows creating a matrix as a 2D array.
$matrix = array(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
This creates a 3×3 matrix with the values 1 to 9. To access individual elements of the matrix, you can use the square bracket notation like this:
echo $matrix[0][0]; // Output: 1
echo $matrix[1][2]; // Output: 6
echo $matrix[2][1]; // Output: 8
In the above example, $matrix[0][0]
gives the value in the first row and first column of the matrix, which is 1. Similarly, $matrix[1][2]
gives the value in the second row and third column of the matrix, which is 6, and so on.
You can also use loops to iterate over the matrix and perform various operations. For example, to print the matrix in a tabular format, you can use nested loops like this:
echo "<table>";
foreach ($matrix as $row) {
echo "<tr>";
foreach ($row as $value) {
echo "<td>" . $value . "</td>";
}
echo "</tr>";
}
echo "</table>";
This will output the matrix as an HTML table:
1 2 3
4 5 6
7 8 9
You can customize the output by using any HTML tags or formatting within the loop.
Further Reading
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
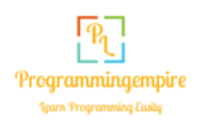