In this article on Comparing constructor injection and setter injection in Spring, I will explain the difference between these two approaches.
In Spring, dependency injection (DI) is a technique that we use to provide the necessary dependencies of an object from outside the object. There are different ways to perform dependency injection, two of which are constructor injection and setter injection.
Basically, constructor injection involves injecting dependencies through a constructor. It means that the dependencies of a class are declared as constructor arguments, and Spring provides the required dependencies when an object of that class is created. This approach ensures that we initialize the dependencies at the time of object creation, making the object ready for use right after the instantiation.
On the other hand, setter injection involves injecting dependencies through setter methods. In this approach, we declare the dependencies as private instance variables, and Spring provides the required dependencies by invoking the corresponding setter methods. Hence, this approach allows for the flexibility of setting the dependencies at runtime and changing them later in the object’s life cycle. The following list shows some differences between the two approaches.
Difference Between Constructor Injection and Setter Injection
- Initialization. In constructor injection, dependencies are initialized during object creation, whereas in setter injection, we initialize dependencies after object creation.
- Immutability. In fact, constructor injection makes the object immutable as the dependencies are set only once during object creation. In contrast, setter injection allows changing the dependencies at runtime. So, it makes the object mutable.
- Readability. Constructor injection makes it easy to read the dependencies of a class because they are declared as arguments in the constructor. In contrast, setter injection requires reading through the entire class to see its dependencies.
- Testing. Constructor injection makes it easy to test the class by passing in mock dependencies through the constructor. In contrast, setter injection requires additional setter methods or reflection to inject mock dependencies during testing.
In general, both approaches have their benefits and drawbacks, and the choice between them depends on the specific use case and requirements. It’s worth noting that Spring supports both constructor injection and setter injection and also offers other forms of dependency injection such as field injection and method injection.
Further Reading
Spring Framework Practice Problems and Their Solutions
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
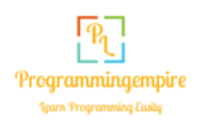