The following program demonstrates how to Compare the Last Digit of Two Numbers in Java.
In order to compare the last digit of numbers that the user has entered, we extract the last digit of each. So, we use the mod (%) operator that gives the remainder after dividing the number by 10.
import java.util.Scanner;
public class Exercise1B
{
public static void main(String[] args) {
int n1, n2, rem1, rem2;
System.out.println("Enter First Number: ");
Scanner scn=new Scanner(System.in);
n1=scn.nextInt();
System.out.println("Enter Second Number: ");
n2=scn.nextInt();
rem1=n1%10;
rem2=n2%10;
if(rem1==rem2)
System.out.println("True");
else
System.out.println("False");
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
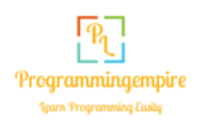