The following article describes what is a companion object in Kotlin, and how is it used.
In Kotlin, a companion object is a special object that is associated with a class. Furthermore, it can be used to define properties and methods that are related to the class but do not depend on a specific instance of the class. Therefore, the companion object is a singleton, which means that there is only one instance of it per class. In order to define a companion object in Kotlin, you use the companion object
keyword inside the class definition. For example.
class MyClass {
companion object {
val someProperty = "This is a property of the companion object"
fun someMethod() {
println("This is a method of the companion object")
}
}
}
As can be seen in the example, we define a companion object for the MyClass
class. Also, the companion object contains a property called someProperty
and a method called someMethod()
. Further, we can access these properties and methods on the class itself, without the need to create an instance of the class. For example.
println(MyClass.someProperty) // prints "This is a property of the companion object"
MyClass.someMethod() // prints "This is a method of the companion object"
The companion object is often used to define factory methods or other utility methods that are related to the class but do not depend on a specific instance of the class. For example, you could use a companion object to define a factory method that creates instances of the class with specific properties.
class Person(val name: String, val age: Int) {
companion object {
fun createDefaultPerson() = Person("John Doe", 30)
}
}
val defaultPerson = Person.createDefaultPerson()
println(defaultPerson.name) // prints "John Doe"
println(defaultPerson.age) // prints "30"
In this example, we define a companion object for the Person
class that contains a factory method called createDefaultPerson()
that creates a Person
instance with default values. This method can be called directly on the Person
class, without the need to create an instance of the class first.
Overall, the companion object is a powerful feature in Kotlin that can help you organize your code and define utility methods and properties that are related to a class but do not depend on a specific instance of the class.
Further Reading
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Data Science
- Data Structures and Algorithms
- Deep Learning
- Django
- Dot Net Framework
- Drones
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Smart City
- Software
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
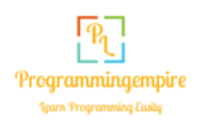