This article describes Checked and Unchecked Exceptions in Java.
- Checked exceptions are exceptions that are checked at compile-time. If a method throws a checked exception, the calling code must either catch the exception or declare it in the method signature using the
throws
keyword. - Unchecked exceptions are exceptions that are not checked at compile-time. These exceptions are typically runtime exceptions and do not need to be declared or caught in the calling code.
Examples of Checked and Unchecked Exceptions in Java
class UncheckedExceptionExample {
public static void main(String[] args) {
int num1 = 10;
int num2 = 0;
System.out.println(num1 / num2);
}
}
class CheckedExceptionExample {
public static void main(String[] args) throws Exception {
File file = new File("test.txt");
Scanner sc = new Scanner(file);
}
}
In the first example, an ArithmeticException
is thrown when dividing num1
by num2
. This is an example of an unchecked exception, because it occurs at runtime and does not need to be declared or caught. The program will simply terminate with an error message.
In the second example, a FileNotFoundException
is thrown when opening the file test.txt
. This is an example of a checked exception, because it occurs at compile time and must be either declared with a throws
clause or caught with a try-catch
block. In this example, the exception is declared with a throws
clause.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
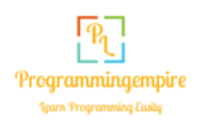