The following code shows how to Check for Prime Number in Java. Prime numbers are those numbers which are divisible only by 1 or itself.
The following code determines whether a randomly generated number is a prime number or not. The function Math.random() generates a random number in the range 0 to 1. In order to find the number in the range 0 to 100, we need to multiply the number returned by Math.random() by 100. Hence, we get a number between 0 and 100. Further, we can start a loop and check whether the given number is divisible by any other number between 2 and half of the given number. In case, it is divisible the number is not prime. Otherwise, the number is prime.
public class Main
{
public static void main(String[] args) {
int a_number, min=0, max=100;
System.out.println("Randomly generating a number in the range ["+min+", "+max+"]: ");
a_number=(int)(Math.random()*100);
System.out.println(a_number);
boolean prime=true;
for(int i=2;i<=a_number/2;i++)
{
if(a_number%i==0)
{
prime=false;
break;
}
}
if(prime)
{
System.out.println(a_number+" is prime!");
}
else{
System.out.println(a_number+" is not prime!");
}
}
}
Output
Randomly generating a number in the range [0, 100]:
96
96 is not prime!
Randomly generating a number in the range [0, 100]:
69
69 is not prime!
Randomly generating a number in the range [0, 100]:
53
53 is prime!
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
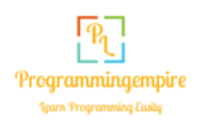