The following code shows how to Check for Armstrong Number in Java. Basically, Armstrong numbers are those which are equal to the sum of each digit raised to the power of number of digits in the number. For example, (i) 21=2 (ii) 153 = 13+53+33 = 1+125+27=153 (iii) 9474 = 94+44+74+44 = 6561 + 256 + 2401 + 256 = 9474 (iv) 54748 = 55 +45 +75 +45 +85 = 3125 + 1024 + 16807 + 1024 + 32768 = 54748.
In order to find whether a number is Armstrong number or not, we follow the steps which are given below.
- At first, find the number of digits in the given number. For this purpose, first we convert the number into a string. After that we can just find the length of the string.
- Initialize a variable that stores sum of digits to 0.
- Next, extract a digit of the number by taking remainder after dividing the number by 10.
- Then, find digit raised to the power length of the number.
- Add this value in the sum.
- Divide the number by 10.
- Repeat steps 2 to 5 until the number becomes 0.
- Compare the sum of digits raised to the power of length with the original number.
- In case both values match, the number is an Armstrong number.
The following code implements above steps.
public class Main
{
public static void main(String[] args) {
int rem, digit_sum=0;
System.out.println("Armstrong Numbers in the range [1, 100000] are...");
for(int n=1;n<=100000;n++)
{
// Find Number of digits in the given number
String number=Integer.toString(n);
int number_len=number.length();
int temp=n;
while(temp!=0){
rem=temp%10;
digit_sum+=Math.round(Math.pow(rem, number_len));
temp=temp/10;
}
if(n==digit_sum)
System.out.println(n);
digit_sum=0;
}
System.out.println();
}
}
Output
Armstrong Numbers in the range [1, 100000] are…
1
2
3
4
5
6
7
8
9
153
370
371
407
1634
8208
9474
54748
92727
93084
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
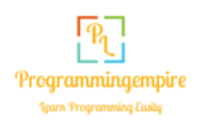