The following program demonstrates how to Arrange Even and Odd Numbers in an Array in Java.
In this program, we have an array of integers. Further, we need to arrange the array elements such that all the even numbers are placed together. Likewise, all the odd numbers in the array should also be placed consecutively. Furthermore, even numbers should shift to the left side. While the odd numbers should be shifted to the right side.
For example, suppose, we have this array, (2,1, 9,7, 8, 5,3,1). So the output should be array=(2, 8, 1, 9, 7, 5, 3, 1).
Another example, input array = (6,1,2,4,5,3,12,9,7,5,1), output array = (6,2,4,12,1,5,3,9,7,5,1).
In order to get the above output, first, we create another array of the same size as the original array. After that, we start a for loop that iterates over all the elements of the original array. When we find an even number, we assign it to the new array. Hence, we get all the even numbers on the left side of the array. Similarly, we repeat this for the odd numbers in another for loop.
public class Exercise10
{
public static void main(String[] args) {
int[] myarray={1, 0, 1, 0, 0, 1, 1};
// int[] myarray={3, 3, 2};
// int[] myarray={2, 2, 2};
// int[] myarray={3, 1, 4, 8, 9, 12, 17, 5, 2, 10, 6, 121, 89, 10};
System.out.println("Array Elements...");
for(int i=0;i<myarray.length;i++)
{
System.out.print(myarray[i]+"\t");
}
int[] tarr=new int[myarray.length];
int j=0;
for(int i=0;i<myarray.length;i++)
{
int x=myarray[i];
if(x%2==0)
{
tarr[j]=x;
j++;
}
}
for(int i=0;i<myarray.length;i++)
{
int x=myarray[i];
if(x%2==1)
{
tarr[j]=x;
j++;
}
}
System.out.println("\nArray Elements After Aranging Even and Odd Numbers...");
for(int i=0;i<tarr.length;i++)
{
System.out.print(tarr[i]+"\t");
}
}
}
Output
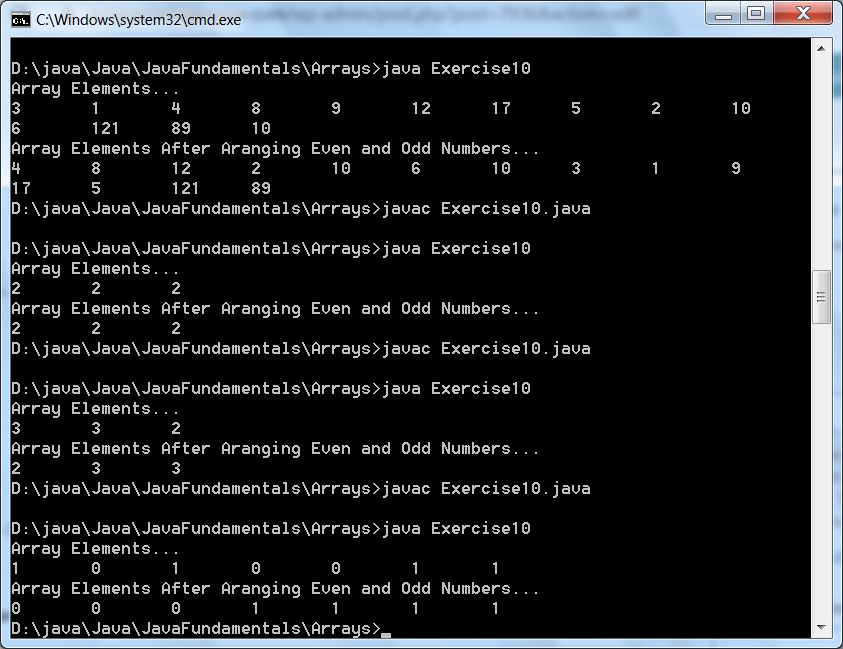
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
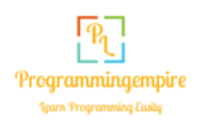