The following program demonstrates An Example of HashSet with User-Defined Objects in Java.
In this program, there is a class called Emp that implements the Comparable interface. So, it defines the compareTo() method that compares the salary of Emp objects and returns an integer accordingly. Further, the HashSet object stores a collection of Emp objects. The contents of the HashSet are displayed using an iterator. As can be seen in the output, HashSet doesn’t maintain any order for its elements.
package com.myhashsets;
import java.util.HashSet;
import java.util.Iterator;
public class HashSetEmpDemo {
public static void main(String[] args) {
// TODO Auto-generated method stub
HashSet<Emp> hash=new HashSet<Emp>();
hash.add(new Emp(11, "A", 12000));
hash.add(new Emp(12, "B", 17700));
hash.add(new Emp(13, "C", 18700));
hash.add(new Emp(14, "D", 14500));
hash.add(new Emp(15, "E", 11400));
hash.add(new Emp(16, "F", 12000));
hash.add(new Emp(17, "G", 16700));
System.out.println("Elements in the HashSet: ");
Iterator it=hash.iterator();
while(it.hasNext())
{
System.out.println(it.next().toString()+" ");
}
}
}
class Emp implements Comparable<Emp>
{
int emp_id;
String ename;
int salary;
public int compareTo(Emp e2)
{
if (salary==e2.getSalary())
return 0;
else
if(salary>e2.getSalary())
return 1;
else
return -1;
}
public Emp() {
super();
}
public Emp(int emp_id, String ename, int salary) {
super();
this.emp_id = emp_id;
this.ename = ename;
this.salary = salary;
}
public int getEmp_id() {
return emp_id;
}
public void setEmp_id(int emp_id) {
this.emp_id = emp_id;
}
public String getEname() {
return ename;
}
public void setEname(String ename) {
this.ename = ename;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
@Override
public String toString() {
return "Employee Details: [emp_id=" + emp_id + ", ename=" + ename + ", salary=" + salary + "]";
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
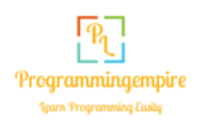