The following program demonstrates A Simple Example of Inheritance in Java.
In the following program, we have a class called Animal. While the class Bird is a subclass of the Animal class. Also, note that the subclass Bird has redefined the methods eat(), and sleep(). Furthermore, it has added its own method fly(). The main() method creates the objects of both subclass and superclass and calls the corresponding methods.
class Animal {
public void eat() {
System.out.println("Animal Eating");
}
public void sleep() {
System.out.println("Animal Sleeping");
}
}
class Bird extends Animal {
public void eat() {
System.out.println("Bird Eating");
}
public void sleep() {
System.out.println("Bird Sleeping");
}
public void fly() {
System.out.println("Bird Flying");
}
}
public class Exercise1 {
public static void main(String[] args) {
Animal animal_ob=new Animal();
Bird bird_ob=new Bird();
animal_ob.eat();
animal_ob.sleep();
bird_ob.eat();
bird_ob.sleep();
bird_ob.fly();
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
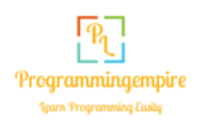