The following code shows a A Simple Example of fetch_assoc() Function in PHP.
Basically, the following example demonstrate how to use the fetch_assoc() function to retrieve the result of a select query. In order to perform some operation on a database table, first you need to connect with the database with specified username, and password. The following code shows the content of the file db.php that establishes a connection with the database.
Since mysqli has both procedural as well as object-oriented interface, we can use either. Here we are using the object-oriented interface. So, in the file db.php we create an object of the mysqli class named con. Also, we need to include this file in the subsequent php script.
db.php
<?php
$host='localhost';
$user='root';
$pass='';
$db='institute';
$con=new mysqli($host, $user, $pass, $db);
if($con->error)
{
die('Error connecting to the database '.$con->error);
}
else
{
echo 'Connected!<br>';
}
?>
The following code shows how to retrieve and display the select query result. So, first we use the require() method to include the file db.php. Now we can use the connection object con. For the select query, the query() function returns a result object that contains the result of the select query. After that we need to call the fetch_assoc() function of the result object in a loop. Acually, the fetch_assoc() function returns a row of the query result. Further, it returns the row as an associative array. Therefore, we can obtain the value of a specific column by specifying the name of the column as the index in the row array. Here in this example, we use the while loop to display all rows of the select query result using the associative array r.
display.php
<?php
require('db.php');
$q='select * from course';
$retrieved_data=$con->query($q);
while($r=$retrieved_data->fetch_assoc())
{
echo 'Course ID: ', $r['course_id'];
echo ', Course Name: ', $r['course_name'];
echo ', Duration: ', $r['duration'];
echo ', Credits: ', $r['credits'];
echo '<br>';
}
$con->close();
?>
Output
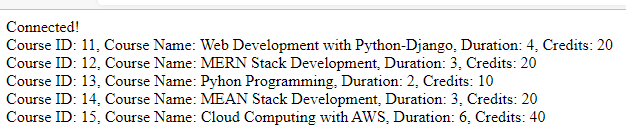
Further Reading
Examples of Array Functions in PHP
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
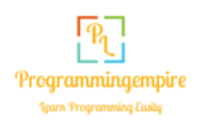