Here are 50+ interview questions along with their answers on Python.
- What is Python, and what are its key features?
Answer: Python is a high-level, interpreted programming language known for its simplicity and readability. Key features include easy syntax, dynamic typing, automatic memory management, and a large standard library.
2. What is the difference between Python 2 and Python 3?
Answer: Python 2 is an older version of Python, while Python 3 is the current and more modern version. Python 3 introduced various syntax changes and improvements, including better Unicode support, print() as a function, and integer division by default.
3. Explain the Global Interpreter Lock (GIL) in Python.
Answer: The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple threads from executing Python code simultaneously. It can limit multi-core CPU performance in Python threads but doesn’t affect multi-processing.
4. How is memory managed in Python?
Answer: Python uses automatic memory management. It has a built-in garbage collector that reclaims memory by deallocating objects with no references.
5. What is PEP 8, and why is it important?
Answer: PEP 8 is the Python Enhancement Proposal that defines the style guide for writing Python code. Following PEP 8 is essential for code readability and consistency.
6. How do you comment out multiple lines of code in Python?
Answer: You can use triple-quotes ('''
or """
) for multi-line comments, but it’s not a recommended practice. The common way is to use the #
character at the beginning of each line.
7. What are Python data types for numbers?
Answer: Python has int (integers), float (floating-point numbers), and complex (complex numbers) data types for representing numbers.
8. How do you swap the values of two variables in Python?
Answer: You can use a temporary variable:
a, b = b, a
9. What is a list comprehension? Provide an example.
Answer: List comprehension is a concise way to create lists in Python. Example:
squares = [x**2 for x in range(10)]
10. Explain the difference between a tuple and a list.
Answer: A tuple is immutable (cannot be changed after creation), while a list is mutable (can be modified). Lists are defined with square brackets [ ]
, and tuples with parentheses ( )
.
11. How do you open and close a file in Python?
Answer: You can use the open()
function to open a file and the close()
method to close it:
file = open('example.txt', 'r') # Open file for reading
# Use file...
file.close()
12. What is a lambda function in Python?
Answer: A lambda function is an anonymous, small, and inline function defined using the lambda
keyword. Example:
add = lambda x, y: x + y
13. Explain the purpose of __init__
method in a Python class.
Answer: __init__
is a constructor method that initializes an object’s attributes when an instance of a class is created.
14. How do you handle exceptions in Python?
Answer: Python provides try
, except
, finally
, and else
blocks for exception handling. For Example.
try:
# Code that may raise an exception
except ExceptionType as e:
# Handle the exception
else:
# Executed if no exception is raised
finally:
# Cleanup code (always executed)
15. What is a generator in Python?
Answer: A generator is a type of iterable that generates values on-the-fly using the yield
keyword, allowing you to iterate over large data sets efficiently.
16. Explain the use of decorators in Python.
Answer: Decorators are functions that modify the behavior of other functions or methods. They are often used for code reuse, logging, and access control.
17. What is a virtual environment in Python, and why is it used?
Answer: A virtual environment is an isolated environment that allows you to manage dependencies and project-specific packages separately from the system-wide Python installation. It helps avoid conflicts and keeps projects self-contained.
18. How do you install external packages in Python?
Answer: You can use the pip
command to install packages from the Python Package Index (PyPI). For example, pip install package_name
.
19. What is the purpose of the if __name__ == "__main__"
statement in a Python script?
Answer: It allows you to check if a Python script is being run as the main program or if it’s being imported as a module. It’s often used to execute code only when the script is run directly.
20. What is the difference between a shallow copy and a deep copy of an object in Python?
Answer: A shallow copy creates a new object but does not recursively copy nested objects, while a deep copy creates a completely independent copy of the object and all objects contained within it.
21. What is a module in Python?
Answer: A module is a Python file containing Python code, functions, and variables that can be imported and reused in other Python scripts.
22. How do you import a module in Python?
Answer: You can use the import
statement to import a module. For example, to import the math
module: import math
.
23. Explain list slicing in Python.
Answer: List slicing is the process of extracting a portion of a list by specifying start, stop, and step values using the list[start:stop:step]
syntax.
24. What is the purpose of the pass
statement in Python?
Answer: The pass
statement is a placeholder that does nothing. It is often used when a statement is syntactically required but no action is needed.
25. How do you iterate over a dictionary in Python?
Answer: You can use a for
loop to iterate over the keys, values, or key-value pairs in a dictionary using methods like keys()
, values()
, or items()
.
26. What are list comprehensions and dictionary comprehensions?
Answer: List comprehensions and dictionary comprehensions are concise ways to create lists and dictionaries, respectively, using a single line of code.
27. Explain the map()
and filter()
functions in Python.
Answer: map()
applies a given function to all items in an iterable and returns an iterator of the results. filter()
filters items in an iterable based on a given function’s criteria.
28. What is the purpose of the super()
function in Python?
Answer: super()
is used to call a method from a parent class in a subclass while providing access to the subclass’s methods and attributes.
29. What is the Global Variable and Local Variable in Python?
Answer: Global variables are declared outside of functions and can be accessed throughout the entire program. Local variables are declared inside functions and have limited scope, existing only within the function.
30. Explain the difference between ==
and is
in Python.
Answer: ==
is used to check if two objects have the same content, while is
is used to check if two objects are the same, i.e., if they occupy the same memory location.
31. What is the purpose of the join()
method for strings in Python?
Answer: The join()
method is used to concatenate a list of strings into a single string, using a specified delimiter.
32. How do you remove duplicates from a list in Python?
Answer: You can remove duplicates from a list by converting it to a set and then back to a list or by using list comprehensions.
33. Explain the purpose of the __str__
and __repr__
methods in Python classes.
Answer: __str__
is used to define the “informal” or user-friendly string representation of an object, while __repr__
defines the “formal” or unambiguous representation, often used for debugging.
34. What is a decorator in Python, and why might you use one?
Answer: A decorator is a function that wraps another function, adding behavior or functionality to it. Decorators are often used for logging, authentication, or modifying function behavior.
35. How can you handle multiple exceptions in a single except
block in Python?
Answer: You can use a tuple of exception types in a single except
block to handle multiple exceptions. For example:
try:
# Code that may raise exceptions
except (ExceptionType1, ExceptionType2) as e:
# Handle exceptions
36. What is the purpose of the enumerate()
function in Python?
Answer: enumerate()
is used to iterate over elements in an iterable while keeping track of their index. It returns tuples containing the index and the item.
37. How do you define and use a class method in Python?
Answer: A class method is defined using the @classmethod
decorator and takes the class itself as its first argument (cls
). It’s often used for factory methods or methods that operate on the class itself, not on instances.
38. Explain the use of the with
statement in Python.
Answer: The with
statement is used to manage resources like files or database connections. It ensures that the resource is properly initialized and released, even if an exception occurs.
39. What is a set in Python, and what is its primary use?
Answer: A set is an unordered collection of unique elements. Its primary use is to perform set operations like union, intersection, and difference.
40. How do you check if a key exists in a dictionary?
Answer: You can use the in
operator to check if a key exists in a dictionary. For example:
if 'key' in my_dict:
# Key exists
41. What is the purpose of the zip()
function in Python?
Answer: The zip()
function combines two or more iterables (e.g., lists or tuples) element-wise, creating an iterator of tuples.
42. Explain the difference between append()
and extend()
methods in Python lists.
Answer: The append()
method adds a single element to the end of a list, while the extend()
method adds all elements from an iterable (e.g., another list) to the end of the list.
43. How do you create and use a Python generator function?
Answer: A generator function is defined like a regular function but contains yield
statements to yield values one at a time. It can be iterated over using a for
loop.
44. What is a docstring in Python?
Answer: A docstring is a string literal used as the first statement in a module, function, class, or method. It serves as documentation to describe the purpose and usage of the code.
45. Explain the purpose of the __init__.py
file in a Python package.
Answer: The __init__.py
file is used to mark a directory as a Python package. It can contain package-level initialization code and is executed when the package is imported.
46. How do you read and write binary files in Python?
Answer: Binary files can be read and written using the rb
(read binary) and wb
(write binary) modes when opening files with open()
.
47. What is the purpose of the json
module in Python, and how do you use it?
Answer: The json
module is used to work with JSON data in Python. It can be used to serialize Python objects into JSON strings and deserialize JSON strings into Python objects.
48. How can you find the length of a string in Python?
Answer: You can use the len()
function to find the length (number of characters) of a string.
49. Explain the use of the join()
method for lists in Python.
Answer: The join()
method is used to concatenate a list of strings into a single string, using a specified delimiter.
50. What is the purpose of the any()
and all()
functions in Python?
Answer: The any()
function returns True
if at least one element in an iterable is True
. The all()
function returns True
if all elements in an iterable are True
.
51. What is the difference between the +=
and append()
operations for lists in Python?
Answer: +=
is used to extend a list by adding elements from another iterable (in-place modification), while append()
adds a single element to the end of a list.
52. How do you create and use a Python virtual environment?
Answer: You can create a virtual environment using the venv
module or the virtualenv
package and activate it using platform-specific commands. It isolates Python environments for different projects.
53. Explain the purpose of the os
module in Python.
Answer: The os
module provides functions for interacting with the operating system, such as file and directory manipulation, environment variable access, and running system commands.
54. What is a lambda function, and when should you use one?
Answer: A lambda function is an anonymous, small, and inline function defined using the lambda
keyword. It’s used for simple operations and is often employed in functional programming and as arguments to higher-order functions like map()
and filter()
.
55. How do you handle file exceptions in Python when opening a file?
Answer: You can use a try
and except
block to catch and handle file-related exceptions, such as FileNotFoundError
and PermissionError
.
56. Explain the purpose of the next()
function in Python iterators.
Answer: The next()
function retrieves the next item from an iterator. It raises StopIteration
if there are no more items.
57. What is the purpose of the assert
statement in Python, and when should it be used?
Answer: The assert
statement is used for debugging and sanity-checking. It tests a condition and raises an AssertionError
if the condition is False
.
58. How do you remove an element by value from a list in Python?
Answer: You can use the remove()
method to remove the first occurrence of a specific value from a list. If the value occurs multiple times, you’ll need to loop and remove them individually.
59. Explain the use of the isinstance()
function in Python.
Answer: isinstance()
is used to check if an object is an instance of a particular class or type. It returns True
if the object is an instance and False
otherwise.
60. What is method overloading in Python, and does Python support it?
Answer: Method overloading, as seen in some other programming languages, is not directly supported in Python. Python allows you to define multiple methods with the same name in a class, but it considers only the latest definition.
Further Reading
How to Perform Dataset Preprocessing in Python?
Spring Framework Practice Problems and Their Solutions
How to Implement Linear Regression from Scratch?
Getting Started with Data Analysis in Python
Wake Up to Better Performance with Hibernate
Data Science in Insurance: Better Decisions, Better Outcomes
Breaking the Mold: Innovative Ways for College Students to Improve Software Development Skills
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
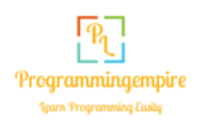