Here are 10 hands-on exercises on list slicing in Python along with their solutions.
Exercise 1: Given a list numbers
, create a new list containing the first three elements.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = numbers[:3]
print(result)
Solution 1: Output: [1, 2, 3]
Exercise 2: Given a list letters
, extract the last two elements.
letters = ['a', 'b', 'c', 'd', 'e']
result = letters[-2:]
print(result)
Solution 2: Output: ['d', 'e']
Exercise 3: Given a list my_list
, create a new list containing every second element, starting from the first element.
my_list = [10, 20, 30, 40, 50, 60, 70, 80, 90]
result = my_list[::2]
print(result)
Solution 3: Output: [10, 30, 50, 70, 90]
Exercise 4: Given a list fruits
, extract a sublist containing the fruits from the third element to the fifth element.
fruits = ['apple', 'banana', 'cherry', 'date', 'fig', 'grape']
result = fruits[2:5]
print(result)
Solution 4: Output: ['cherry', 'date', 'fig']
Exercise 5: Given a list numbers
, extract all even-indexed elements.
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
result = numbers[::2]
print(result)
Solution 5: Output: [0, 2, 4, 6, 8]
Exercise 6: Given a list colors
, create a new list containing the last three elements in reverse order.
colors = ['red', 'green', 'blue', 'yellow', 'purple']
result = colors[-1:-4:-1]
print(result)
Solution 6: Output: ['purple', 'yellow', 'blue']
Exercise 7: Given a list nums
, extract a sublist containing the elements from index 2 to the end in steps of 2.
nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = nums[2::2]
print(result)
Solution 7: Output: [3, 5, 7, 9]
Exercise 8: Given a list words
, extract a sublist containing the first two elements and the last two elements.
words = ['apple', 'banana', 'cherry', 'date']
result = words[:2] + words[-2:]
print(result)
Solution 8: Output: ['apple', 'banana', 'cherry', 'date']
Exercise 9: Given a list numbers
, extract a sublist containing all elements except the first and last elements.
numbers = [10, 20, 30, 40, 50]
result = numbers[1:-1]
print(result)
Solution 9: Output: [20, 30, 40]
Exercise 10: Given a list characters
, create a new list containing every third element, starting from the second element.
characters = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i']
result = characters[1::3]
print(result)
Solution 10: Output: ['b', 'e', 'h']
These exercises should help you practice and become more comfortable with list slicing in Python.
Further Reading
How to Perform Dataset Preprocessing in Python?
Spring Framework Practice Problems and Their Solutions
How to Implement Linear Regression from Scratch?
How to Use Generators in Python?
Getting Started with Data Analysis in Python
Wake Up to Better Performance with Hibernate
Data Science in Insurance: Better Decisions, Better Outcomes
How to Use Decorators in Python?
Common Ways to Create Web Applications in Python
Breaking the Mold: Innovative Ways for College Students to Improve Software Development Skills
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
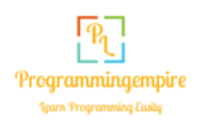