The following code example demonstrates how to Find Grade Using Switch Statement in JSP.
Basically, the following program shows how to create a dynamic web application in JSP to find the grade according to the marks entered by the user using the switch statement.
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Grade Calculator</title>
</head>
<body>
<h2>Grade Calculator</h2>
<form action="GradeCalculator.jsp" method="post">
Enter Marks: <input type="text" name="marks"> <br> <br>
<input type="submit" value="Submit">
</form>
<%
int marks = Integer.parseInt(request.getParameter("marks"));
char grade;
switch (marks / 10) {
case 10:
case 9:
grade = 'A';
break;
case 8:
grade = 'B';
break;
case 7:
grade = 'C';
break;
case 6:
grade = 'D';
break;
default:
grade = 'F';
}
out.println("<br><br>Your Grade is: " + grade);
%>
</body>
</html>
In this program, we first create a form with an input field for the user to enter the marks. The form submits the marks to the same JSP page using the post
method. When the form is submitted, the JSP page retrieves the marks using request.getParameter("marks")
and converts it to an integer using Integer.parseInt()
.
Next, we use a switch statement to calculate the grade based on the marks. The switch statement divides the marks by 10 and checks the value of the result to determine the grade. If the marks are between 90 and 100, the grade is ‘A’, between 80 and 89 it’s ‘B’, between 70 and 79 it’s ‘C’, between 60 and 69 it’s ‘D’, and otherwise it’s ‘F’.
Finally, we use out.println()
to print the grade. Note that the JSP code is enclosed within <%
and %>
tags.
Further Reading
Spring Framework Practice Problems and Their Solutions
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
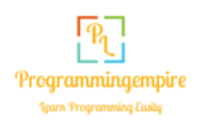