In this article, I will discuss Method And Function Overloading In Python.
Basically, in Python, method overloading is not supported. Because the language follows a dynamic, duck-typing approach. In other words, the same method name can be used for different types of objects. However, we can achieve function overloading through default arguments, variable-length arguments (*args, **kwargs), and function annotations.
Difference Between Method And Function Overloading In Python
As a matter of fact, in Python, there’s no method overloading. In contrast, we can achieve function overloading. The following list specifies the ways to perform function overloading.
- Default arguments. In fact, by providing a default value for one or more parameters, a single function can handle multiple input cases. Hence, we get overloaded functions.
- *args and **kwargs. Another way to achieve function overloading is to use variable-length arguments. These arguments allow a function to accept a variable number of positional and keyword arguments.
- Function annotations. Moreover, we can achieve function overloading by using Function annotations. Basically, annotations provide a way to give extra information about a function’s arguments and return values.
To summarize, function overloading in Python is achieved through default arguments, *args and **kwargs, and function annotations, whereas method overloading is not supported.
Example of Function Overloading in Python
The following example demonstrates function overloading in python.
#!/usr/bin/env python
# coding: utf-8
# In[3]:
class Sum():
def add(x,y):
return x+y
def add(x,y,z):
return x+y+z
#print(Sum.add(5,6))
print(Sum.add(5,6,7))
# In[7]:
class Sum():
def add(x,y,z=None):
if z is None:
return x+y
else:
x+y+z
print(Sum.add(5,6))
# In[23]:
class Sum():
def add(x,y,z=None):
if z is None:
return x+y
else:
return x+y+z
print(Sum.add(5,5,10))
# In[34]:
class Distance():
def __init__(self,f=0,i=0):
self.feet=f
self.inches=i
def __add__(self,o):
temp=Distance()
temp.feet=self.feet+o.feet
temp.inches=self.inches+o.inches
if temp.inches>12:
temp.inches-=12
temp.feet+=1
return temp
def __gt__(self,o):
if self.feet>o.feet:
return True
elif self.feet<o.feet:
return False
elif self.feet== o.feet and self.inches> o.inches:
return True
else:
return False
def __isub__(self,o):
#self.feet-=o.feet
#self.inches-=o.inches first method--
#return self
if self.inches<o.inches:
self.inches+=12
self.feet-=1
self.feet-=o.feet
self.inches-=o.inches
return self
o1=Distance(20,3)
o2=Distance(10,4)
result=o1+o2
if(o1>o2):
print("__gt__--> Object 1 is greater!")
else:
print("__gt__--> Object 2 is greater!")
o1-=o2
print("__isub__-->",o1.feet," ",o1.inches)
print("__add__-->",result.feet," ",result.inches)
# In[35]:
class A():
def process(self):
print("in class A")
class B():
def process(self):
print("in class B")
class C(B,A):
def process(self):
print("in class C")
c1=C()
c1.process()
print(C.mro())
# In[36]:
class A():
def process(self):
print("in class A")
class B():
def process(self):
print("in class B")
class C(A,B):
def process(self):
print("in class C")
c1=C()
c1.process()
print(C.mro())
# In[42]:
class company():
def company_info(c_name,c_location):
def __init__(self,obj):
print("google","USA")
def display():
pass #glt
class employee(company):
def emp_info(e_name,e_salary):
super().company_info
#print("ABC",120000)
e=employee()
e.emp_info("ABC",120000)
print(e)
# In[47]:
class company():
def __init__(self,c_name,c_loc):
self.c_name=c_name
self.c_loc=c_loc
def display(self):
print("Company name:",self.c_name)
print("Company location:",self.c_loc)
class employee(company):
def __init__(self,c_name,c_loc,e_name,e_sal):
super().__init__(c_name,c_loc):
self.e_name=e_name
self.e_sal=e_sal
def display(self):
print("Employee name:",self.e_name)
print("Employee Sal:",self.e_sal)
class employee(company):
e=employee("google","usa","ABC",120000)
e.display
# In[ ]:
Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
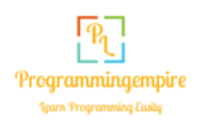