In this blog, I will explain How to Create a Random Quote Generator With StreamLit.
Streamlit is an open-source Python library designed for creating web applications with minimal effort. It’s particularly well-suited for data scientists, engineers, and developers who want to build interactive and data-driven web applications quickly.
Install Streamlit
If you haven’t already, you need to install Streamlit. You can install it using pip:
pip install streamlit
Create a Python Script
Create a Python script (e.g., random_quote_generator.py
) and open it in your favorite code editor.
Import Libraries
In your Python script, import the necessary libraries.
import streamlit as st
import random
Create a List of Quotes
Define a list of quotes that your generator will choose from. You can use quotes related to inspiration, motivation, or any topic you prefer.
quotes = [
"The only way to do great work is to love what you do. - Steve Jobs",
"Believe you can, and you're halfway there. -Theodore Roosevelt",
"Don't watch the clock; do what it does. Keep going. - Sam Levenson",
"Your time is limited, don't waste it living someone else's life. - Steve Jobs",
"The future belongs to those who believe in the beauty of their dreams. - Eleanor Roosevelt"
]
Create the Streamlit Web App
Now, let’s create the Streamlit web app that will display a random quote each time the user clicks a button.
# Set the title and page layout
st.title("Random Quote Generator")
st.sidebar.title("About")
st.sidebar.info("This is a simple Streamlit app that generates random quotes.")
# Create a button to generate a random quote
if st.button("Generate Random Quote"):
random_quote = random.choice(quotes)
st.write(random_quote)
The following script shows the complete code.
import streamlit as st
import random
# List of quotes
quotes = [
"The only way to do great work is to love what you do. - Steve Jobs",
"Believe you can, and you're halfway there. -Theodore Roosevelt",
"Don't watch the clock; do what it does. Keep going. - Sam Levenson",
"Your time is limited, don't waste it living someone else's life. - Steve Jobs",
"The future belongs to those who believe in the beauty of their dreams. - Eleanor Roosevelt"
]
# Streamlit web app
st.title("Random Quote Generator")
st.sidebar.title("About")
st.sidebar.info("This is a simple Streamlit app that generates random quotes.")
if st.button("Generate Random Quote"):
random_quote = random.choice(quotes)
st.write(random_quote)
Run the App
Save your Python script and run the Streamlit app using the following command in your terminal.
streamlit run random_quote_generator.py
Interact with the App
A new tab will open in your web browser with your random quote generator. Click the “Generate Random Quote” button, and you’ll see a random quote displayed on the screen each time you click the button.
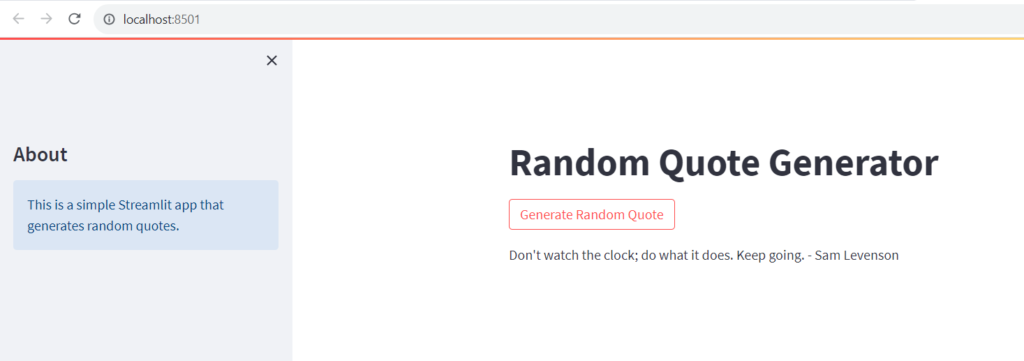
That’s it! You’ve created a simple random quote generator using Streamlit. You can further customize the app’s appearance and functionality to fit your preferences. You can also expand the list of quotes to include more options.
Further Reading
10 Simple Project Ideas on Extended Reality
Examples of Array Functions in PHP
10 Simple Project Ideas on Digital Trust
10 Simple Project Ideas on Datafication
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selections in a Database Table in PHP
PHP Projects for Undergraduate Students
Further Reading
10 Simple Project Ideas on Extended Reality
Examples of Array Functions in PHP
10 Simple Project Ideas on Digital Trust
10 Simple Project Ideas on Datafication
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selections in a Database Table in PHP
PHP Projects for Undergraduate Students
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- Extended Reality
- Flutter and Dart
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML