In this article, I will explain the concept of Linear Regression With One Variable. Also, I will discuss some examples of linear regression in python.
Basically, Linear Regression with One Variable is a simple statistical model that we use to understand the relationship between a single independent variable and a dependent variable. So, in this type of regression, the dependent variable is modeled as a linear function of the independent variable, represented by a line. The goal is to find the line that best fits the data.
The line is represented by the equation y = b0 + b1 * x, where b0 and b1 are coefficients that need to be estimated from the data. b0 is the intercept, representing the value of y when x is zero. b1 is the slope, representing the change in y for a unit change in x.
Once the coefficients are estimated, the model can be used to make predictions for new data by plugging in the values of the independent variable. The line can be used to explain the relationship between the variables, including the strength and direction of the relationship.
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
# Generating the data
np.random.seed(0)
x = np.linspace(0, 10, 20)
y = 2 * x + np.random.randn(20)
# Plotting the data
plt.scatter(x, y)
plt.xlabel('Independent Variable')
plt.ylabel('Dependent Variable')
plt.show()
# Reshaping the data
x = x.reshape(-1, 1)
# Fitting the linear regression model
reg = LinearRegression().fit(x, y)
# Predicting the dependent variable
y_pred = reg.predict(x)
# Plotting the regression line
plt.scatter(x, y)
plt.plot(x, y_pred, color='red')
plt.xlabel('Independent Variable')
plt.ylabel('Dependent Variable')
plt.show()
In this example, we generate some random data with an underlying linear relationship. We plot the data to visualize the relationship. Then, we fit a linear regression model using scikit-learn
‘s LinearRegression
class and make predictions using the predict
method. Finally, we plot the data along with the regression line to see how well the model fits the data.
Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
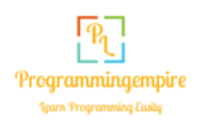