In this article on Illustrating Dynamic Binding with an Example in c#, I will explain the concept of dynamic binding in C#.
Brief Introduction of Dynamic Binding with an Example in c#
Dynamic binding in C# refers to the process of linking method calls to the actual methods at runtime, instead of at compile-time. This allows for greater flexibility in software design, as the type of object being called is determined at runtime, instead of being fixed at compile-time.
Here is an example of dynamic binding in C#:
using System;
class Shape {
public virtual void Draw() {
Console.WriteLine("Drawing a Shape");
}
}
class Circle : Shape {
public override void Draw() {
Console.WriteLine("Drawing a Circle");
}
}
class Square : Shape {
public override void Draw() {
Console.WriteLine("Drawing a Square");
}
}
class Program {
static void Main(string[] args) {
Shape shape = new Shape();
Shape circle = new Circle();
Shape square = new Square();
DrawShape(shape);
DrawShape(circle);
DrawShape(square);
Console.ReadLine();
}
static void DrawShape(Shape shape) {
shape.Draw();
}
}
In this example, the DrawShape
method takes a Shape
object as a parameter. However, at runtime, it could be a Shape
, Circle
, or Square
object. The actual type of the object being passed to the DrawShape
method is determined at runtime, and the appropriate Draw
method is called, demonstrating dynamic binding in C#.
Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
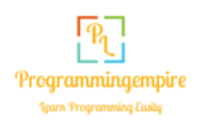