In this blog, I will explain How to Create a Calculator Using StreamLit.
Creating a simple calculator using Streamlit is a great way to demonstrate how user input and interaction can be handled. In this example, I’ll show you how to build a basic calculator that performs addition, subtraction, multiplication, and division. You can then expand on this by adding more functionality or styling.
At first, import the Streamlit library. After that, create the user interface for the calculator using Streamlit’s widgets. You’ll need input fields for the numbers, radio buttons for selecting the operation, and a button to calculate the result.
import streamlit as st
st.title("Calculator")
# Input fields for two numbers
num1 = st.number_input("Enter the first number", value=0.0)
num2 = st.number_input("Enter the second number", value=0.0)
# Radio buttons for selecting the operation
operation = st.radio("Select an operation", ("Addition", "Subtraction", "Multiplication", "Division"))
# Button to calculate the result
if st.button("Calculate"):
if operation == "Addition":
result = num1 + num2
elif operation == "Subtraction":
result = num1 - num2
elif operation == "Multiplication":
result = num1 * num2
elif operation == "Division":
if num2 == 0:
result = "Cannot divide by zero"
else:
result = num1 / num2
st.write(f"Result: {result}")
When you run the app, a new tab will open in your web browser with the calculator. Users can input two numbers, select an operation, and click the “Calculate” button to see the result.
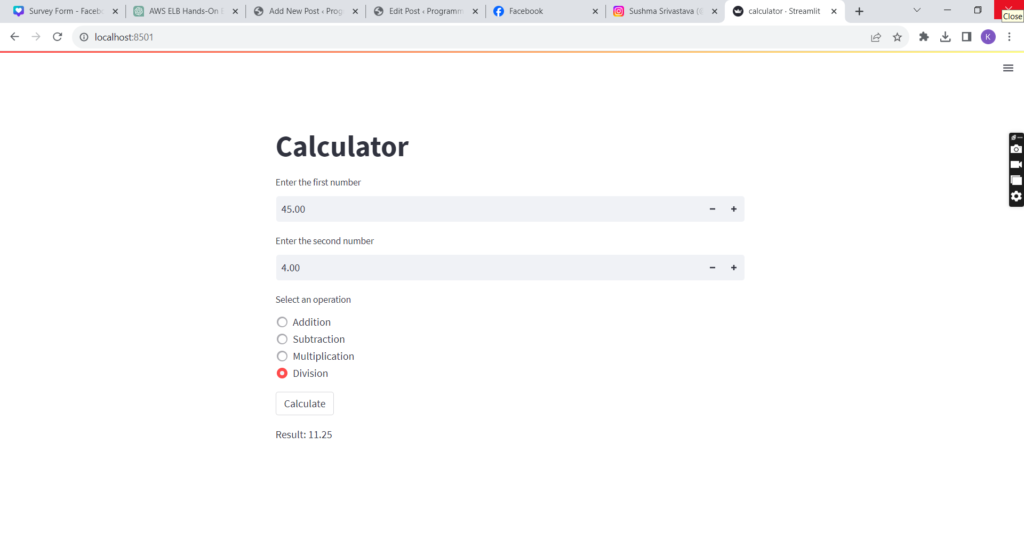
That’s it! You’ve created a simple calculator using Streamlit. You can expand on this by adding more operations, error handling, and advanced features like memory functions or scientific calculations.
Further Reading
10 Simple Project Ideas on Extended Reality
How to Create a Random Quote Generator With StreamLit?
Examples of Array Functions in PHP
How to Create a Survey Form for a Workshop Using StreamLit?
10 Simple Project Ideas on Digital Trust
How to Create a Currency Converter Using StreamLit?
10 Simple Project Ideas on Datafication
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selections in a Database Table in PHP
PHP Projects for Undergraduate Students
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML