In this blog, I will explain How to Create a To-Do List Using Streamlit.
Creating a to-do list application using Streamlit is a great way to build a simple interactive task manager. In this example, I’ll guide you through creating a basic to-do list that allows users to add, edit, and mark tasks as completed. You can then extend this by adding features like task priorities, due dates, and more.
Now, create the user interface for the to-do list using Streamlit’s widgets. You’ll need input fields for adding tasks, a list to display tasks, and buttons for marking tasks as completed and deleting tasks.
import streamlit as st
st.title("To-Do List")
# Input field for adding tasks
task = st.text_input("Add a task")
# Initialize session state to store tasks
if 'tasks' not in st.session_state:
st.session_state.tasks = []
# Button to add a task
if st.button("Add Task"):
if task:
st.session_state.tasks.append({"task": task, "completed": False})
task = ""
# Checkbox to mark all tasks as completed
if st.checkbox("Mark All"):
for t in st.session_state.tasks:
t["completed"] = True
# Checkbox to show completed tasks
show_completed = st.checkbox("Show Completed Tasks")
if show_completed:
tasks_to_display = st.session_state.tasks
else:
tasks_to_display = [t for t in st.session_state.tasks if not t["completed"]]
# Display the list of tasks with checkboxes
st.write("### Tasks")
if tasks_to_display:
for i, t in enumerate(tasks_to_display, start=1):
task = t["task"]
completed = t["completed"]
t["completed"] = st.checkbox(f"{i}. {task}", value=completed)
else:
st.write("No tasks added")
# Button to clear completed tasks
if st.button("Clear Completed Tasks"):
st.session_state.tasks = [t for t in st.session_state.tasks if not t["completed"]]
# Button to clear all tasks
if st.button("Clear All Tasks"):
st.session_state.tasks = []
With this code, you can mark all tasks as completed using a checkbox, show or hide completed tasks, clear completed tasks, and clear all tasks. The to-do list is updated based on your interactions with these new functionalities.
When you run the App, a new tab will open in your web browser with the to-do list. Users can input tasks, mark tasks as completed, and clear completed or all tasks.

That’s it! You’ve created a basic to-do list using Streamlit. You can enhance it by adding features like task priorities, due dates, or task persistence by saving tasks to a database or a file.
Further Reading
How to Perform Dataset Preprocessing in Python?
Spring Framework Practice Problems and Their Solutions
How to Implement Linear Regression from Scratch?
How to Use Generators in Python?
How to Use Technology for Stress Relief?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
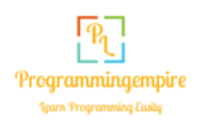