concat()
The following code shows that we have three arrays. Further, we create these arrays by initialising, as well as taking input from the user. Also, we call the concat() method with the first arrays and taking rest of the two arrays as parameters. The output shows that a single large arrays is created.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=Array(3);
var arr2=[1,2,3,4];
var arr3=Array(2);
arr3[0]='abc';
arr3[1]=1.5;
for(var i=0;i<arr1.length;i++)
{
var x=prompt("Enter array element: ");
arr1[i]=Number(x);
}
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
document.write("<br/>Array concatenation...<br/>");
arr4=arr1.concat(arr2, arr3);
for(var i=0;i<arr4.length;i++)
document.write(arr4[i]+" ");
</script>
</head>
<body>
</body>
</html>
Output
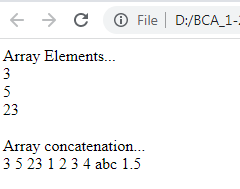
join()
Basically, we use join() method to join elements of an arrays in JavaScript using a separator. The following code shows that the join() method is used to join the elements using a separator ‘-‘.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=Array(3);
for(var i=0;i<arr1.length;i++)
{
var x=prompt("Enter array element: ");
arr1[i]=Number(x);
}
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
document.write("<br/>Array join...<br/>");
var str=arr1.join("-");
document.write(str);
</script>
</head>
<body>
</body>
</html>
Output
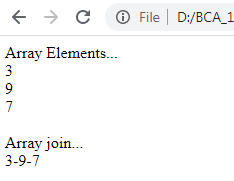
reverse()
The following shows the use of reverse() method. In order to reverse the elements of array, we call this method using the array object.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=Array(3);
for(var i=0;i<arr1.length;i++)
{
var x=prompt("Enter array element: ");
arr1[i]=Number(x);
}
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
document.write("<br/>Reversing array...<br/>");
arr1.reverse();
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
</script>
</head>
<body>
</body>
</html>
Output

sort()
The following code shows that the use of sort() method to sort the elements of an array.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=Array(3);
for(var i=0;i<arr1.length;i++)
{
var x=prompt("Enter array element: ");
arr1[i]=Number(x);
}
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
document.write("<br/>Sorting array...<br/>");
arr1.sort();
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
</script>
</head>
<body>
</body>
</html>
Output
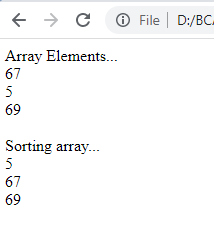
indexOf()
The following code shows how to use the indexOf() function. Basically, this function returns the index of the first occurrence of the specified element. Therefore, in the following, the firtst call to this method returns 2 since this the index of first occurrence of the element 9. However, the next call returns 7. Since it is the first occurrence of 9 beginning from the index 3. The second parameter of the indexOf() function specifies the index from where the searching should start. Similarly, the third call returns -1 since the element 90 is not present. Likewise, the last call returns 11 since searching starts from the index 10.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=[8,1,9, 12, 1, 3, 1, 9, 14, 16, 12, 9];
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
document.write("Using indexOf()....<br/>");
var a=arr1.indexOf(9);
document.writeln(a);
var a=arr1.indexOf(9, 3);
document.writeln(a);
var a=arr1.indexOf(90);
document.writeln(a);
var a=arr1.indexOf(9, 10);
document.writeln(a);
</script>
</head>
<body>
</body>
</html>
Output
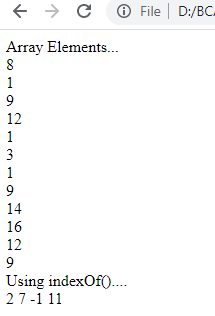
lastIndexOf()
The following code shows the examples of lastIndexOf() function. This function begins searching from the last index. Further, the second parameter indicates the index from where the searching should begin in backward direction.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=[8,1,9, 12, 1, 3, 1, 9, 14, 16, 12, 9];
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
document.write("Using lastIndexOf()....<br/>");
var a=arr1.lastIndexOf(9);
document.writeln(a);
var a=arr1.lastIndexOf(9, 3);
document.writeln(a);
var a=arr1.lastIndexOf(90);
document.writeln(a);
var a=arr1.lastIndexOf(9, 10);
document.writeln(a);
</script>
</head>
<body>
</body>
</html>
Output
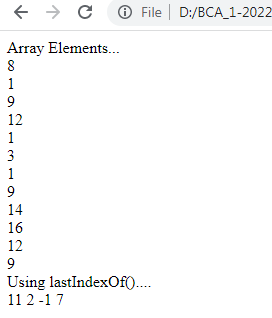
push()
In order to insert an element in the last we use the push() method.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=[8,1,9, 12, 1, 3, 1, 9, 14, 16, 12, 9];
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
arr1.push(100);
document.write("Array Elements after push() called...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
</script>
</head>
<body>
</body>
</html>
Output
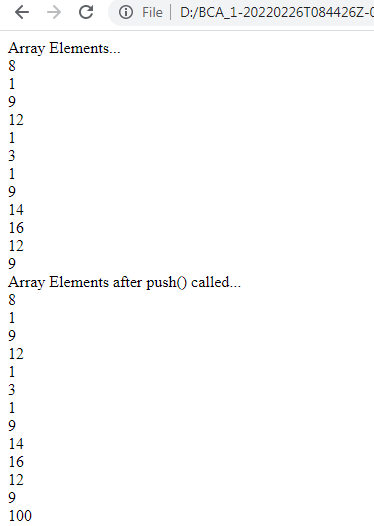
pop()
The following code shows how to use the pop() method in order to return and remove the last element.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=[8,1,9, 12, 1, 3, 1, 9, 14, 16, 12, 9];
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
var x=arr1.pop();
document.writeln("Element Returned by pop() method: "+x);
document.write("<br/>Array Elements after pop() called...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
</script>
</head>
<body>
</body>
</html>
Output
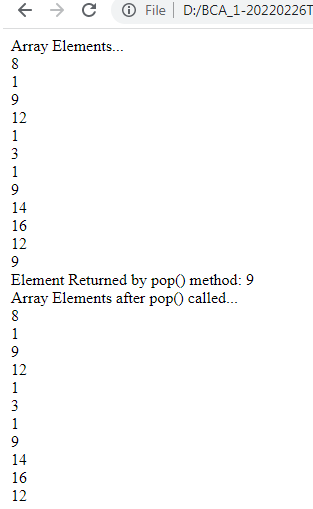