In this blog, I will explain How to Create a Survey Form for a Workshop Using StreamLit.
Creating a survey form for a workshop using Streamlit is a straightforward process. You can use Streamlit’s widgets to create input fields for gathering responses and then store or analyze the collected data. Here’s a step-by-step guide on how to create a simple survey form with Streamlit.
Install Streamlit
If you haven’t already, install Streamlit using pip.
pip install streamlit
Create a Python Script
Create a Python script (e.g., workshop_survey.py
) and open it in your code editor.
Import Libraries
In your Python script, import the necessary libraries.
import streamlit as st
Build the Survey Form
Define the survey questions and input fields using Streamlit’s widgets. You can use text inputs, radio buttons, checkboxes, and text areas to gather responses.
Here’s an example of a simple survey form with three questions.
st.title("Workshop Survey Form")
# Question 1
question1 = st.text_input("1. What is your name?")
# Question 2
question2 = st.radio("2. Did you find the workshop informative?", ("Yes", "No"))
# Question 3
question3 = st.text_area("3. Please share your feedback on the workshop (optional)")
Capture Responses
You can capture the responses and perform actions with them. For this example, we’ll print the responses to the console.
if st.button("Submit"):
st.write("Thank you for participating in the survey.")
st.write("Survey Responses:")
st.write(f"Name: {question1}")
st.write(f"Found Workshop Informative: {question2}")
st.write(f"Feedback: {question3}")
Run the App
Save your Python script and run the Streamlit app using the following command in your terminal.
streamlit run workshop_survey.py

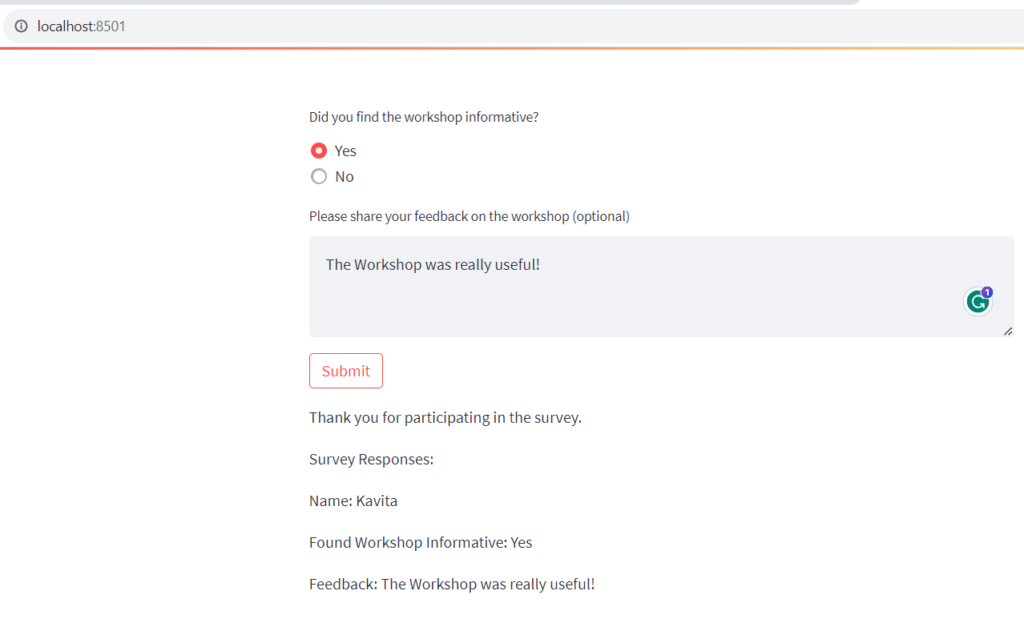
Interact with the Survey
A new tab will open in your web browser, displaying the workshop survey form. Users can input their responses and click the “Submit” button to see the collected responses.
That’s it! You’ve created a simple workshop survey form using Streamlit. You can customize the survey questions, add more widgets, and store or analyze the responses as needed for your specific use case.
Further Reading
10 Simple Project Ideas on Extended Reality
How to Create a Random Quote Generator With StreamLit?
Examples of Array Functions in PHP
10 Simple Project Ideas on Digital Trust
10 Simple Project Ideas on Datafication
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selections in a Database Table in PHP
PHP Projects for Undergraduate Students
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML