The following article presents some Basic Code Examples Using Flask API.
Here are some basic programs in Flask that you can start with.
- Display Message. A simple program that returns a text message on the home page.
from flask import Flask
app = Flask(__name__)
@app.route('/')
def programmingempire():
return 'Learn on programmingempire.com!'
if __name__ == '__main__':
app.run(debug=True)
- Dynamic Routing. A program that uses dynamic routing to accept input from the user in the URL and display it on the page.
from flask import Flask
app = Flask(__name__)
@app.route('/<name>')
def hello_name(name):
return f'Hello, {name}!'
if __name__ == '__main__':
app.run(debug=True)
- Form Submission. A program that uses an HTML form to accept user input and display it on the page.
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
@app.route('/submit', methods=['POST'])
def submit():
name = request.form.get('name')
return f'Hello, {name}!'
if __name__ == '__main__':
app.run(debug=True)
- RESTful API. A program that creates a simple RESTful API using Flask.
from flask import Flask, jsonify, request
app = Flask(__name__)
students = [
{'id': 1, 'name': 'Alice'},
{'id': 2, 'name': 'Bob'},
{'id': 3, 'name': 'Charlie'}
]
@app.route('/students')
def get_students():
return jsonify(students)
@app.route('/students/<int:id>')
def get_student(id):
student = next(filter(lambda s: s['id'] == id, students), None)
if student:
return jsonify(student)
else:
return jsonify({'message': 'Student not found'})
if __name__ == '__main__':
app.run(debug=True)
These are just a few examples of basic programs in Flask. With Flask’s flexible and easy-to-use framework, you can create a wide range of web applications and APIs to suit your needs.
Further Reading
How to Start Working with Flask API?
20 Project Ideas Using Flask API for College Students
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
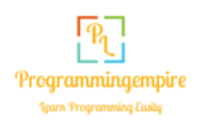