The following ArrayList Exercise in Java will help you to work with the ArrayList collection class.
- Create an ArrayList in Java and add the days of the week. Also, display the ArrayList.
- Define a class Emp with the following fields – emp_id, name, salary, gender, department, and email_id. Also, define the corresponding constructors, and getter and setter methods. Further, override the toString() method to display the details of the employee. Now create another class EmpList, that has an ArrayList as its data member. In this class, define two methods – addEmp(Emp ob), and deleteEmp(int eid) that adds and removes an Emp object in the list. Also, create methods to display minimum salary, maximum salary, average salary, and search an employee using the given emp_id. Finally, test the program using the main() method.
- Write a program that creates an ArrayList of strings and displays its elements using an iterator.
- Create an ArrayList to add elements of all the numeric types like int, float, double, long, and byte and display its elements using an iterator. However, it should not contain elements of any other type.
- Perform all the tasks given in Exercise 2 using LinkedList.
- Also, perform all the tasks given in Exercise 4 using LinkedList.
- Implement Exercise 2 using the Vector class.
- Also, implement Exercise 1 using LinkedList and Vector. (i) Using Linked List (ii) Using Vector
- When do we use Iterator? In order to demonstrate the use of iterator and enumeration, create a Vector of the Emp objects.
- Further, modify Exercise 5 to display the first and the last object.
- Write a program to demonstrate the iterations over multiple collections in parallel.
- In order to create an ArrayList of Companies, create a Company class that contains the company_id, company_name, and an ArrayList of Emp objects as its attributes. Display the details of companies using an iterator.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
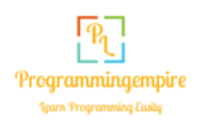