In this article, I will discuss how to Find the Longest Sequence of Negative Numbers and Find its Sum in Java.
The following code example shows the use of a nested loop to find the longest sequence of negative number. The problem is stated as follows:
We have an array of integers containing both positive and negative numbers. Also, the number 0 is considered as positive in this problem. So, our goal is to first find the longest sequence of consecutive negative numbers. Further, the sum of this sequence should be computed. In case, there are more than one such sequences, then all of them should be summed up.
The following example describes the problem and its output.
Array: [12, 0, 9, 10, 5, -5, -8, 23, 1, 9, -1,-1,-1,-1,8, 0, 6, -2,-3,-2, 8]
Sequences of Negative numbers are (i) -5, -8 (ii) -1, -1, -1, -1 (iii) -2, -3, -2. Also, their lengths are 2, 4, and 3 respectively. So, the longest length here is 4 and the corresponding sum is -4. Hence, the output is -4.
Another example, let the Array be [-3, -9, -10, -12, -5, -10]. Here, we have only one such sequence with the length of 6. So, the output is the sum of elements in this sequence, that is, -49.
When, there is no negative number, in the array, the output should be 1. So, for the array = [2, 1, 6, 3, 5, 0, 7], the program should display 1.
When, there are more than one such sequences (of longest lengths), the output should be the of all elements belonging to these sequences. For example, if the array is [-2, 3, 1, 6, -9, -8, -1, 4, 6, 3, -1, -1, -1, 7, 1, -2, -3, -7, 1, 2, -1, 6, -10, -20], the sequences with negative numbers are (i) -2 (ii) -9, -8, -1 (iii) -1, -1, -1 (iv) -2, -3, -7 (v) -1 (vi) -10, -20. Further, the lengths of these sequences are 1, 3, 3, 3, 1, and 2 respectively. So, the longest sequence has length 3. Moreover, there are 3 such sequences. So, we sum up all the elements belonging to them. Hence, the output is -33.
Program to Find the Longest Sequence of Negative Numbers and Find its Sum in Java
The following code provides the solution using a nested loop.
public class Sequences
{
public static void main(String[] args)
{
int[] arr1={1,2,-1,-3,5, -2,-1, -9, 6, 1, 10, 2};
int[] arr2={-3, -9, -10, -12, -5, -10};
int[] arr3={2,1, 6, 3, 5, 0, 7};
int[] arr4={-2, 3, 1, 6, -9, -8, -1, 4, 6, 3, -1, -1, -1, 7, 1, -2,-3,-7, 1, 2, -1, 6, -10, -20};
System.out.println(findSum(arr1));
System.out.println(findSum(arr2));
System.out.println(findSum(arr3));
System.out.println(findSum(arr4));
}
static int findSum(int[] arr)
{
int maxlen=0, maxsum=0, totalsum=0;
int i=0, j=0;
int positives=0;
int tlen=0, tsum=0;
int len=arr.length;
while(i<len)
{
if(arr[i]>=0)
{
i++;
positives++;
}
else
{
j=i;
while(j<len && arr[j]<0)
{
tlen++;
tsum+=arr[j];
j++;
}
if(maxlen<tlen)
{
maxlen=tlen;
maxsum=tsum;
}
else
if(maxlen==tlen)
{
maxsum+=tsum;
}
tlen=0;
tsum=0;
i=j;
}
}
totalsum=maxsum;
if(positives==len)
totalsum=1;
return totalsum;
}
}
Output
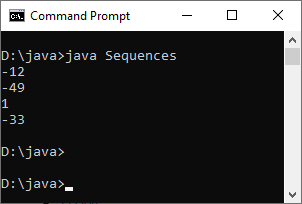
Further Reading
Understanding Enterprise Java Beans
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML