In this article on A Mini Project on JDBC Operation, the use of HDBC API in Java is explained through an application.
To begin with, create the table Exam. The following list provides the details of the columns in the exam table.
- user_id: varchar(100)
- password: varchar(100)
- user_type: varchar(100)
- incorrect_attempts: Number(2)
- lock_status: Number(2)
- user_name: varchar(100)
The following operations need to be performed on the above table. In this example, we are using Oracle 11g for the database. After creating the table, insert some records into the table. Therefore, we use the following commands.
create table Exam(user_id varchar(100) primary key, password varchar(100), user_type varchar(100), incorrect_attempts number(2), lock_status number(2), user_name varchar(100));
insert into Exam(user_id, user_name, password, user_type, incorrect_attempts, lock_status) values('1101', 'A', '123', 'student', 0, 0);
insert into Exam(user_id, user_name, password, user_type, incorrect_attempts, lock_status) values('1102', 'B', '345', 'student', 0, 0);
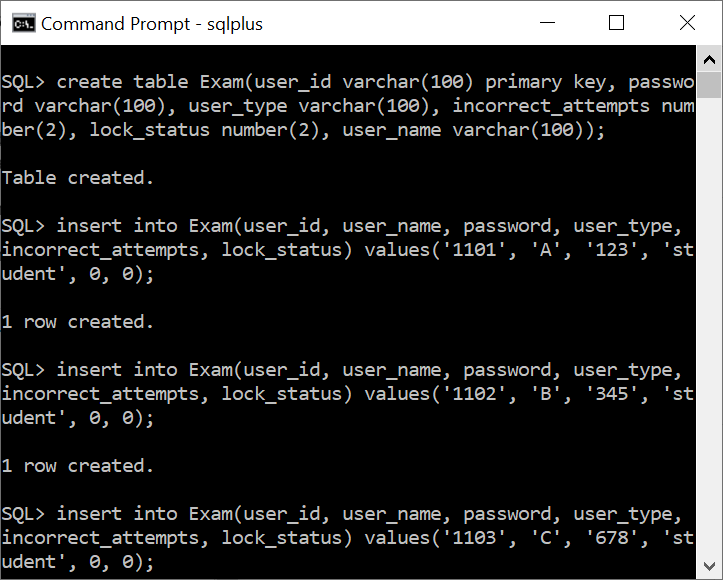
- Get a Connection object by calling the getDBConnection() method.
- Using this Connection object, retrieve the user_type and display it.
- In order to retrieve and display the values of the user_type column, call the getUserType() method from the main() method.
- Create a method called String getIncorrectAttempts(String user_id) and perform the following tasks: (i) Obtain a Connection object by calling the getConnection() method. (ii) Retrieve the incorrect attempts. If the incorrect attempts are 0, then display the message “No Incorrect Attempts”. However, if its value is 1, then display “One Time”. Otherwise, display the message “Incorrect Attempts Exceeded”. For this purpose, create a method called getIncorrectAttempts() and call this method from the main() method.
Updating the Records
- Another method String changeUserType(String user_type) should perform the following tasks: (i) Obtain the Connection object. (ii) For the specified user_id, update the user_type to Admin. If the update is successful, display the message “Update Success”. Otherwise, display “Update Failed”. (iii) In order to perform these tasks, create a method changeUserType() and call it from the main() method.
- Create a method called getLockStatus() and perform the following tasks. (i) Obtain a Connection object. (ii) For the lock status 0, count the number of rows. (iii) In order to perform this create a method getLockStatus() and call it from the main() method.
- Another method String changeName(String user_id, String newName) should perform the following tasks. (i) Obtain a Connection object and change the user_name for the given user_id (ii) Also, create a method changeName() and call it from the main() method. (iii) If it results in success, the changeName() method should return “Success”. Otherwise, it should return “Failed”.
- Similarly, create the changePassword() method with the following signature.
String changePassword(String user_id, String newPassword)
Inserting Records
The following methods need to be created for inserting records.
Further Reading
Understanding Enterprise Java Beans
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML