Here I will discuss Python Program for Linear Regression With Multiple Variables.
The following program demonstrates how to use Linear Regression for multiple variables.
import numpy as np
import pandas as pd
from sklearn.linear_model import LinearRegression
# Load data into a pandas DataFrame
data = pd.read_csv('data.csv')
# Split the data into independent and dependent variables
X = data.iloc[:, :-1]
y = data.iloc[:, -1]
# Fit a linear regression model to the data
model = LinearRegression()
model.fit(X, y)
# Print the coefficients of the model
print('Coefficients:', model.coef_)
# Predict the target values for new data
new_data = np.array([[3, 5, 7]])
prediction = model.predict(new_data)
print('Prediction:', prediction)
This program uses the LinearRegression
class from the sklearn
library to fit a linear regression model to the data. The data is loaded into a pandas
DataFrame, and the independent and dependent variables are split using the iloc
method. The fit
method is used to fit the model to the data, and the coef_
attribute is used to print the coefficients of the model. The predict
method is used to make predictions for new data.
Note that this is just a basic example, and you may need to modify or add additional code to handle missing values, outlier detection, or feature scaling.
Further Reading
Examples of OpenCV Library in Python
A Brief Introduction of Pandas Library in Python
A Brief Tutorial on NumPy in Python
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
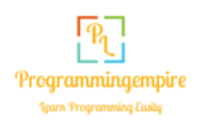