Programmingempire
This article demonstrates a program Using Numbers As Command Line Arguments in Java. To begin with, let us first understand what is a command-line argument. In general, a command-line argument is passed to the program at the time of executing the command to run the program. In other words, when we run a Java program at the console, we can also specify the arguments separated by a space character. The main() method within the class receives these arguments in an array of type String.
Benefits of Using Command Line Arguments
In fact, there is no restriction on the total number of arguments that we can pass at the command line. Moreover, the type of these arguments is String. Therefore, we can easily convert these arguments to any other type such as numeric type. Further, the command-line arguments provide us with a convenient way to check the behavior of the program.
A Simple Example of Using Command Line Arguments
public class Main
{
public static void main(String[] myarguments) {
String s1, s2, s3;
s1=myarguments[0];
s2=myarguments[1];
s3=myarguments[2];
System.out.println("Command Line Arguments: "+s1+", "+s2+", "+s3);
}
}
The following command runs the above program.
javac Main.java
java Main Apple Mango Banana
Output:
Command Line Arguments: Apple, Mango, Banana
Another Example of Using Numbers As Command Line Arguments in Java
public class Main
{
public static void main(String[] myarguments) {
int p, q, r;
p=Integer.parseInt(myarguments[0]);
q=Integer.parseInt(myarguments[1]);
r=Integer.parseInt(myarguments[2]);
if((p>q) && (p>r))
System.out.println(p + " is largest!");
else if((q>p) && (q>r))
System.out.println(q + " is largest!");
else
System.out.println(p + " is largest!");
}
}
Likewise, we use the following commands to compile and run the above program.
javac Main.java
java Main 78 87 4
Output:
87 is largest!
Hence, in the above program, we specify the arguments in the same manner as before. However, once we receive the arguments in the main() method as string values, we can convert them to the value of the appropriate type. Similarly, we can pass the arguments of any other type. For example, the following program demonstrates the computing area of a circle using command line arguments. As can be seen, here we need an input value of type double. Therefore, we can convert the input string received as a command line argument to double.
public class Main
{
public static void main(String[] myarguments) {
final double pi=3.14;
double radius, area;
radius=Double.parseDouble(myarguments[0]);
System.out.println("Area of the Circle is "+pi*radius*radius);
}
}
Running the program:
javac Main.java
java Main 7.983
Output:

- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
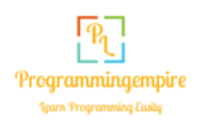