Programmingempire
This article demonstrates Method-Overloading Using Java. Earlier, I have explained how to create user-defined methods. Accordingly, a class can have user-defined methods which are all uniquely named. In other words, in a particular class, each method has a unique name. However, this rule has an exception. In other words, a class may allow two or more methods to have the same name as long as their signatures differ. Basically, this concept is called Method Overloading. In fact, Method Overloading allows two or more methods in a class to have the same name but different signatures.
What Comprises the Signature of a Method?
Specifically, a method signature comprises three elements. In brief, these three elements are – number of parameters, the data type of parameters, and the order of parameters. Accordingly, the return type of a method is not included in its signature. Therefore, overloading methods involve altering the above three elements for the methods having the same name. As an illustration, see the following examples.
At first, the two overloaded methods are defined with the same name – f1. As can be seen, both methods have two parameters of different data types.
int f1(int a, int b){
// Statements
}
int f1(double a, double b){
// Statements
}
Another example of overloaded methods is shown below. As can be seen, both of the methods are having the same name – f2. Also, both of these methods have two parameters of int, and double type respectively. However, the order of parameters is different in both methods. Therefore, the method f2() can be overloaded in this way.
int f2(int a, double b){
// Statements
}
int f2(double a, int b){
// Statements
}
As an illustration, the following overloaded methods have a different number of parameters. Hence, these methods can be overloaded.
int f3(int a, int b){
// Statements
}
int f3(int b){
// Statements
}
Example of Method Overloading Using Java
The following example demonstrates the overloaded methods to find the minimum of certain numbers. Accordingly, we can define the overloaded methods to find the minimum of two numbers, the minimum of three numbers, and the minimum of an array elements.
package MethodOverloading;
public class OverloadingExample {
public static void main(String[] args) {
int p=67, q=57;
System.out.println("Minimum of "+p+" and "+q+" is "+findMin(p,q));
int x=46, y=58, z=39;
System.out.println("Minimum of "+x+", "+y+" and "+z+" is "+findMin(x, y, z));
int[] a1= {89, 12, 9, 32, 7, 34, 97, 15};
System.out.println("Array Elements...");
for(int m:a1)
System.out.print(m+" ");
System.out.println("nMinimum of array elements: "+findMin(a1));
}
public static int findMin(int a, int b) {
if (a<b)
return a;
else
return b;
}
public static int findMin(int a, int b, int c) {
if((a<b) && (a<c))
return a;
else
if((b<a) && (b<c))
return b;
return c;
}
public static int findMin(int[] arr) {
int min=arr[0];
for(int i=1;i<arr.length;i++)
{
if(min>arr[i])
min=arr[i];
}
return min;
}
}
Output

- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
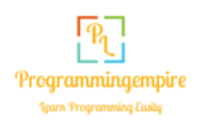