The following article describes How to perform rendering a model in the Django Admin interface with an example.
To render a model in the Django Admin interface, you can create an Admin class for the model and register it with the admin site. Here’s an example of how to do this for a complaint-tracking system.
- Define your model in models.py
from django.db import models
class Complaint(models.Model):
my_name = models.CharField(max_length=250)
description = models.TextField()
date_filed = models.DateTimeField(auto_now_add=True)
resolved = models.BooleanField(default=False)
def __str__(self):
return self.my_name
- Create an Admin class for your model in admin.py
from django.contrib import admin
from .models import Complaint
class ComplaintAdmin(admin.ModelAdmin):
list_display = ('my_name', 'date_filed', 'resolved')
list_filter = ('resolved',)
search_fields = ('My_name', 'description')
admin.site.register(Complaint, ComplaintAdmin)
In the above code, ComplaintAdmin
is an admin class for the Complaint
model. The list_display
attribute specifies the fields to display in the admin list view, list_filter
specifies the filters to include on the right-hand side of the list view, and search_fields
specifies the fields to search when using the search box.
- Access the admin interface
Navigate to http://localhost:8000/admin
. Once logged in, you’ll be able to view, add, edit and delete Complaints.
Note: You will need to make sure that your app is included in your project’s INSTALLED_APPS
setting.
That’s it! You now have a Django Admin interface for your Complaint model.
Further Reading
Introduction to Django Framework and its Features
Examples of Array Functions in PHP
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
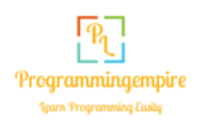