In this article, I will explain How to Create and Use CRUD Class-Based Views using an example application.
Basically, we will create a CRUD Class based view in Django for a shopping list application. The following code shows the implementation of a CRUD (Create, Retrieve, Update, Delete) class-based view in Django for a shopping list application.
from django.views.generic import ListView, CreateView, UpdateView, DeleteView
from django.urls import reverse_lazy
from .models import ShoppingList
class ShoppingListView(ListView):
model = ShoppingList
template_name = 'shopping_list.html'
class ShoppingCreateView(CreateView):
model = ShoppingList
fields = ['date', 'items']
template_name = 'shopping_form.html'
success_url = reverse_lazy('shopping_list')
class ShoppingUpdateView(UpdateView):
model = ShoppingList
fields = ['date', 'items']
template_name = 'shopping_form.html'
success_url = reverse_lazy('shopping_list')
class ShoppingDeleteView(DeleteView):
model = ShoppingList
template_name = 'shopping_confirm_delete.html'
success_url = reverse_lazy('shopping_list')
In the above code, we have created four views, namely ShoppingListView
, ShoppingCreateView
, ShoppingUpdateView
, and ShoppingDeleteView
.
ShoppingListView
is a ListView that displays all the ShoppingList objects. It uses a template named shopping_list.html
.
ShoppingCreateView
is a CreateView that allows the user to create a new ShoppingList object. It uses a template named shopping_form.html
. Once the object is created, the user is redirected to the ShoppingListView
.
ShoppingUpdateView
is a UpdateView that allows the user to update an existing ShoppingList object. It uses the same shopping_form.html
template as ShoppingCreateView
. Once the object is updated, the user is redirected to the ShoppingListView
.
ShoppingDeleteView
is a DeleteView that allows the user to delete an existing ShoppingList object. It uses a template named shopping_confirm_delete.html
. Once the object is deleted, the user is redirected to the ShoppingListView
.
You will also need to create a model for the ShoppingList object, which should look something like this.
from django.db import models
class ShoppingList(models.Model):
date = models.DateField()
items = models.TextField()
def __str__(self):
return f'Shopping List for {self.date}'
In this model, we have defined two fields: date
, which is a DateField, and items
, which is a TextField. The __str__
method is used to display the object in a human-readable format.
Make sure to add the views to your urls.py
file, like this.
from django.urls import path
from .views import ShoppingListView, ShoppingCreateView, ShoppingUpdateView, ShoppingDeleteView
urlpatterns = [
path('', ShoppingListView.as_view(), name='shopping_list'),
path('create/', ShoppingCreateView.as_view(), name='shopping_create'),
path('update/<int:pk>/', ShoppingUpdateView.as_view(), name='shopping_update'),
path('delete/<int:pk>/', ShoppingDeleteView.as_view(), name='shopping_delete'),
]
This creates four URLs: one for the ShoppingListView
, one for the ShoppingCreateView
, one for the ShoppingUpdateView
, and one for the ShoppingDeleteView
. The URLs use the names we gave them earlier (shopping_list
, shopping_create
, shopping_update
, and shopping_delete
). The pk
parameter is used to identify the specific object to update or delete.
Using the CRUD Class-Based Views Application
Once you have created the ShoppingList
model and added the CRUD views and URLs to your Django project, here’s how you can use the application.
- Visit the
shopping_list
URL to view all the ShoppingList objects. Initially, there won’t be any objects. - Visit the
shopping_create
URL to create a new ShoppingList object. Enter the date (e.g. “2023-04-24”) and the list of items (e.g. “bag, fruits, vegetables, bucket”) and submit the form. You will be redirected back to theshopping_list
URL and the newly created object will be displayed. - To update an existing object, click on the “Update” link next to the object on the
shopping_list
page. This will take you to theshopping_update
URL, where you can edit the date and items and submit the form. You will be redirected back to theshopping_list
URL and the updated object will be displayed. - To delete an existing object, click on the “Delete” link next to the object on the
shopping_list
page. This will take you to theshopping_delete
URL, where you can confirm the deletion by clicking the “Delete” button. You will be redirected back to theshopping_list
URL and the deleted object will no longer be displayed.
Sample Code for Using the Application
# Import the ShoppingList model
from .models import ShoppingList
# Step 1: View all the ShoppingList objects
def shopping_list(request):
shopping_lists = ShoppingList.objects.all()
context = {'shopping_lists': shopping_lists}
return render(request, 'shopping_list.html', context)
# Step 2: Create a new ShoppingList object
def shopping_create(request):
if request.method == 'POST':
date = request.POST['date']
items = request.POST['items']
shopping_list = ShoppingList(date=date, items=items)
shopping_list.save()
return redirect('shopping_list')
else:
return render(request, 'shopping_form.html')
# Step 3: Update an existing ShoppingList object
def shopping_update(request, pk):
shopping_list = ShoppingList.objects.get(id=pk)
if request.method == 'POST':
shopping_list.date = request.POST['date']
shopping_list.items = request.POST['items']
shopping_list.save()
return redirect('shopping_list')
else:
context = {'shopping_list': shopping_list}
return render(request, 'shopping_form.html', context)
# Step 4: Delete an existing ShoppingList object
def shopping_delete(request, pk):
shopping_list = ShoppingList.objects.get(id=pk)
if request.method == 'POST':
shopping_list.delete()
return redirect('shopping_list')
else:
context = {'shopping_list': shopping_list}
return render(request, 'shopping_confirm_delete.html', context)
To add the URLs for these views, you can use Django’s urls.py
file.
from django.urls import path
from . import views
urlpatterns = [
path('shopping-list/', views.shopping_list, name='shopping_list'),
path('shopping-create/', views.shopping_create, name='shopping_create'),
path('shopping-update/<int:pk>/', views.shopping_update, name='shopping_update'),
path('shopping-delete/<int:pk>/', views.shopping_delete, name='shopping_delete'),
]
Make sure to include this file in your project’s urls.py
file using the include
function.
from django.urls import include, path
urlpatterns = [
# ... other URL patterns ...
path('shopping/', include('shopping.urls')),
]
Assuming you have also created the templates shopping_list.html
, shopping_form.html
, and shopping_confirm_delete.html
, you should now be able to use the shopping list application.
That’s it! You can use these views to create, retrieve, update, and delete ShoppingList objects in your Django application.
Further Reading
Introduction to Django Framework and its Features
Examples of Array Functions in PHP
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
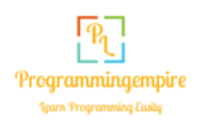