In this article, I will explain Django Views in details.
What is a Django View?
To begin with, we need to understand that what is actually a Django View. Basically, a view in Django is a Python function that handles HTTP requests and returns a response in a web application built using the Django framework. Further, a view is responsible for processing user input, performing any necessary calculations, and returning a response in the form of an HTML page, JSON data, or any other content type.
In Django, we typically define views in the views.py file of an app. In fact, these view are mapped to URLs using URL patterns. Furthermore, a view function can receive data from the request, process it, and use the Django template system to render a response. After that, it returns the response to the user.
Also, the Views in Django are reusable, modular, and testable. So, it makes it easy to write and maintain large web applications. Furthermore, they are a key component of the Model-View-Template (MVT) architectural pattern used in Django web development.
Example
The following code shows a simple example of a Django view.
from django.shortcuts import render
from django.http import HttpResponse
def home_view(request):
return render(request, 'home.html')
def about_view(request):
return HttpResponse("<h1>About Page</h1>")
The above code defines two views: home_view
and about_view
.
home_view
uses the render
shortcut to return a template response, where request
is the incoming request object and 'home.html'
is the name of the template to be rendered.
about_view
returns a simple HTTP response using the HttpResponse
class. It returns an HTML string with a header tag.
Note: The views in this example are not mapped to any URL yet. To use these views, you need to create a URL pattern in your urls.py
file and map it to the corresponding view function.
Further Reading
Introduction to Django Framework and its Features
Creating a Simple App in Django
What are the Important Concepts in Django?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
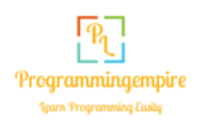