The following post provides 20+ Programming Problems on Control Statements in PHP. Also, the solutions are available on respective links.
- Print numbers from 45 to 225 using a for loop.
- Then, print even numbers from 7 to 120 using a for loop.
- Also, print odd numbers from 9 to 155 using a while loop.
- Find the sum of all even numbers from 12 to 230 using a for loop.
- Also, find the sum of all odd numbers from 56 to 981 using a while loop.
- Check if a number is even or odd.
- Also, check if a number is prime or not.
- Print the Fibonacci series up to a given number.
- Find the factorial of a given number using a for loop.
- Also, find the factorial of a given number using a while loop.
- Check a string for palindrome.
- Print the multiplication table of a given number.
- Compute the GCD of two numbers using any loop.
- Also, find the LCM of two numbers using any loop.
- Print the ASCII values of all characters from A to Z.
- Perform decimal to binary conversion.
- Also, perform binary to decimal conversion.l.
- Reverse a given string.
- Find the largest and smallest numbers in an array.
- Check if an array is sorted in ascending order.
- Also, check if an array is sorted in descending order.
- Remove all the negative numbers from an array.
- Find the average of all the elements in an array.
- From an integer array, find the second-largest element.
- Display the Fibonacci series up to 10 terms.
- Calculate the sum of the digits of a given number.
- Reverse a given number.
- Print the sum of the first 65 natural numbers.
- Also, print the sum of the first 45 even numbers.
- Find the largest among the three given numbers.
- Also, find the smallest among the three given numbers.
- Check if a given string is a palindrome or not.
- Display, from a specific string,
- how many vowels are there?
- Also, display, how many words are there.
- Print the ASCII value of all characters.
- Find the length of the longest word.
- Remove all whitespace from a given string.
- Convert a given string to uppercase.
- Also, convert a given string to lowercase.
- Replace all occurrences of a given substring in a string with another substring.
- Display the first n prime numbers.
- Compare three numbers to find the largest using the ternary operator.
- Display the Fibonacci series up to a given value of the term.
- Print the multiplication table of a given number.
- Also, print the ASCII values of all the characters in a string.
- From an integer array, get the second largest element.
- Likewise, get the second smallest element.
- Sort an array of integers in ascending order using bubble sort.
- Also, sort an array of integers in descending order using selection sort.
- Find the sum of all even numbers in an array.
- Also, find the sum of all odd numbers in an array.
- Then, find the average of an array of numbers.
- Also, find the mode of an array of numbers.
- Find the median of an array of numbers.
- Print a pattern of stars in a pyramid shape.
- Also, print a pattern of numbers in a pyramid shape.
- Then, print a pattern of alphabets in a pyramid shape.
- Further, print a pattern of stars in a right-angled triangle shape.
- Then, print a pattern of numbers in a right-angled triangle shape.
- Next, print a pattern of alphabets in a right-angled triangle shape.
- Print a pattern of stars in an inverted pyramid shape.
- Also, print a pattern of numbers in an inverted pyramid shape.
- Next, print a pattern of alphabets in an inverted pyramid shape.
- Print a pattern of stars in a diamond shape.
- Then, print a pattern of numbers in a diamond shape.
- Next, print a pattern of alphabets in a diamond shape.
- Also, print a pattern of stars in a hollow square shape.
- Print a pattern of numbers in a hollow square shape.
- Then, print a pattern of alphabets in a hollow square shape.
- Next, print a pattern of stars in a hollow triangle shape.
- Also, print a pattern of numbers in a hollow triangle shape.
- Display a pattern of alphabets in a hollow triangle shape.
- Print a pattern of stars in a hollow diamond shape.
- Then, print a pattern of numbers in a hollow diamond shape.
- Next, print a pattern of alphabets in a hollow diamond shape.
- Also, print a pattern of stars in a rectangle shape.
- Display a pattern of numbers in a rectangle shape.
- Print a pattern of alphabets in a rectangle shape.
- Then, print a pattern of stars in a hollow rectangle shape.
- Next, print a pattern of numbers in a hollow rectangle shape.
- Print a pattern of alphabets in a hollow rectangle shape.
Further Reading
10+ File Handling Problems in PHP
Pattern Display Problems in PHP
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
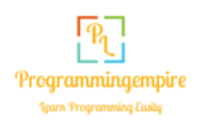