The following post provides several Pattern Display Problems in PHP with their solutions.
- Print a pattern of stars in a pyramid shape:
<?php
for($i=1; $i<=5; $i++){
for($j=1; $j<=5-$i; $j++){
echo " ";
}
for($k=1; $k<=$i; $k++){
echo "* ";
}
echo "<br/>";
}
?>
Output:
*
* *
* * *
* * * *
* * * * *
- Print a pattern of numbers in a pyramid shape:
<?php
for($i=1; $i<=5; $i++){
for($j=1; $j<=5-$i; $j++){
echo " ";
}
for($k=1; $k<=$i; $k++){
echo $k." ";
}
echo "<br/>";
}
?>
Output:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
- Print a pattern of alphabets in a pyramid shape:
<?php
$start = ord('A');
$end = ord('E');
for($i=$start; $i<=$end; $i++){
for($j=$start; $j<=$end-$i+$start-1; $j++){
echo " ";
}
for($k=$start; $k<=$i; $k++){
echo chr($k)." ";
}
echo "<br/>";
}
?>
Output:
A
A B
A B C
A B C D
A B C D E
- Print a pattern of stars in a right-angled triangle shape:
<?php
for($i=1; $i<=5; $i++){
for($j=1; $j<=$i; $j++){
echo "* ";
}
echo "<br/>";
}
?>
Output:
*
* *
* * *
* * * *
* * * * *
- Print a pattern of numbers in a right-angled triangle shape:
<?php
for($i=1; $i<=5; $i++){
for($j=1; $j<=$i; $j++){
echo $j." ";
}
echo "<br/>";
}
?>
Output:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
- Print a pattern of alphabets in a right-angled triangle shape:
<?php
$start = ord('A');
$end = ord('E');
for($i=$start; $i<=$end; $i++){
for($j=$start; $j<=$i; $j++){
echo chr($j)." ";
}
echo "<br/>";
}
?>
Output:
A
A B
A B C
A B C D
A B C D E
- Print a pattern of stars in an inverted pyramid shape:
<?php
for($i=5; $i>=1; $i--){
for($j=1; $j<=5-$i; $j++){
echo " ";
}
for($k=1; $k<=$i; $k++){
Further Reading
10+ File Handling Problems in PHP
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
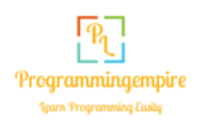