In this article on the Examples of Built-in Functions of Arrays in PHP, I will explain various built-in array functions in PHP using code examples.
The Following Section Provides Some Examples of Built-in Functions of Arrays in PHP
array_chunk()
In order to split an array into chunks of the specified size, we use this function. Basically, this function takes an array and the size of the chunk as parameters. It creates chunks of a given size. However, the last array contains only the remaining elements.
<?php
//Example of array_chunk()
$arr=array(1,4,5,7,12,-9,33,78,1,90,56,88, 11, 12, 13, 99, 110, 543);
echo 'Total elements in array: '.count($arr);
echo '<br>Array Elements...';
foreach($arr as $a)
echo $a.' ';
echo '<br>Splitting the above array in chunks of size 4...<br>';
$chunks=array_chunk($arr, 4);
foreach($chunks as $c)
{
print_r($c);
echo '<br>';
}
?>
Output
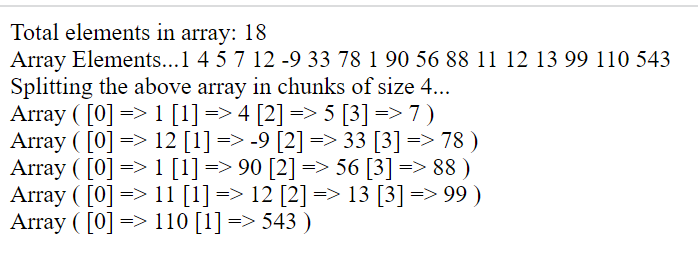
array_column()
For the purpose of retrieving the elements of any specified column, we can use the array_chunk() function. For instance, in the previous example, we create an array of chunks of size 4. So, to display the elements from a specified column name of all the arrays we can use this function. In other words, the name of the column needs to be specified as the second parameter. Therefore, the first of the following example displays all elements of column ‘0’, and so on.
<?php
echo '<br><br>Printing elements of column [0]...<br>';
$zerocolumn=array_column($chunks, '0');
print_r($zerocolumn);
echo '<br><br>Printing elements of column [1]...<br>';
$onecolumn=array_column($chunks, '1');
print_r($onecolumn);
echo '<br><br>Printing elements of column [2]...<br>';
$twocolumn=array_column($chunks, '2');
print_r($twocolumn);
echo '<br><br>Printing elements of column [3]...<br>';
$threecolumn=array_column($chunks, '3');
print_r($threecolumn);
?>
Output

list()
In order to create the variables from the elements of an array, we use the list() function. So, each element of the array is assigned to a variable specified as a parameter starting from the first element. In case, there are more variables than the number of elements in the array, an error occurs.
<?php
$arr=array('ab', 'bc', 'cd', 'de', 'ed', 'fg', 'gh');
list($x, $y, $z)=$arr;
echo $x.' '.$y.' '.$z.'<br>';
list($p)=$arr;
echo $p.'<br>';
list($a, $b, $c, $d, $e, $f, $g)=$arr;
echo $a.' '.$b.' '.$c.' '.$d.' '.$e.' '.$f.' '.$g.'<br>';
// list($a, $b, $c, $d, $e, $f, $g, $h)=$arr; Error
// echo $a.' '.$b.' '.$c.' '.$d.' '.$e.' '.$f.' '.$g.' '.$h.'<br>';
?>
Output
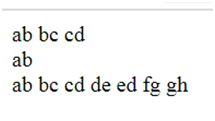
array_slice()
The following PHP script shows an example of the array_slice() method. As can be seen, this method creates a slice of an array starting from the specified index and containing the specified number of elements. Accordingly, the first parameter of the function is an array, the second one represents the starting element, and the last parameter represents the count of elements in the slice.
<?php
$arr=array('ab', 'bc', 'cd', 'de', 'ed', 'fg', 'gh');
$arr1=array_slice($arr, 3, 4);
print_r($arr);
echo '<br>';
print_r($arr1);
echo '<br>';
$arr2=array_slice($arr, 3, 4, true);
print_r($arr);
echo '<br>';
print_r($arr2);
echo '<br>';
?>
Output
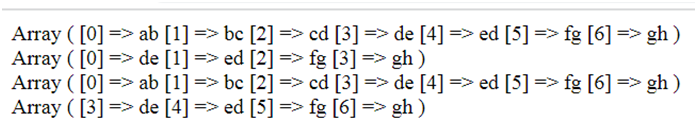
array_splice()
Similarly, the array_splice() method takes a part of the array. However, it removes elements from the array and replaces the removed part with new elements. The following PHP script shows an example of the array_splice() method.
<?php
echo 'Original Array...<br>';
$arr1=array('ab', 'bc', 'cd', 'de', 'ed', 'fg', 'gh');
$arr2=array('xx', 'yy', 'zz');
print_r($arr1);
echo '<br>';
print('Array retrieved after splice....<br>');
print_r(array_splice($arr1, 3, 2));
print('<br>Original array after splice...<br>');
print_r($arr1);
echo '<br>Original Array...<br>';
$arr1=array('ab', 'bc', 'cd', 'de', 'ed', 'fg', 'gh');
print_r($arr1);
echo '<br>';
print('Array retrieved after splice....<br>');
print_r(array_splice($arr1, 3, 2, $arr2));
echo '<br>';
print('<br>Original array after splice...<br>');
print_r($arr1);
?>
Output

More Examples of Built-in Functions of Arrays in PHP
array_sum()
Likewise, the array_sum() method computes the sum of array elements.
<?php
echo 'Original Array...<br>';
$arr1=array(3, 1, 90, -55, 23, 12, 80);
$sum=array_sum($arr1);
print_r($arr1);
echo '<br>Sum of lements: '.$sum;
?>
Output

array_product()
Similarly, the array_product() method computes the product of array elements.
<?php
echo 'Original Array...<br>';
$arr1=array(3, 6, -2, -5, 2, 7, 15);
$product=array_product($arr1);
print_r($arr1);
echo '<br>Product of elements: '.$product;
?>
Output

array_reverse()
The following code shows an example of the array_reverse() method that returns the reverse of the given array.
<?php
echo 'Original Array...<br>';
$arr1=array(3, 6, -2, -5, 2, 7, 15);
$arr2=array_reverse($arr1);
print_r($arr1);
echo '<br>Reversed Array...<br> ';
print_r($arr2);
?>
Output

Examples of Built-in Functions of Arrays in PHP – Sorting Functions
PHP offers various built-in functions for sorting arrays. The following section provides examples on sort(), rsort(), asort(), arsort(), ksort(), krsort().
sort() and resort()
Basically, we use the sort() method to sort an indexed array in the ascending order. Whereas, the rsort() method sorts the elements of the array in descending order. However, both of these methods sort the values of the array.
<?php
echo 'Original Array...<br>';
$arr1=array(3, 6, -2, -5, 2, 7, 15);
print_r($arr1);
echo '<br>Sorting an indexed array in ascending order...<br> ';
sort($arr1);
print_r($arr1);
echo '<br>';
echo '<br>Sorting an indexed array in descending order...<br> ';
rsort($arr1);
print_r($arr1);
?>
Output
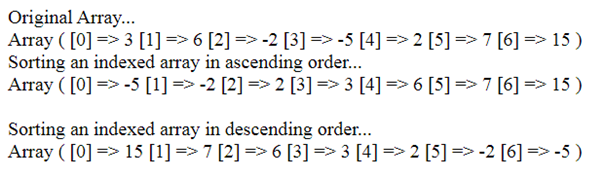
asort() and arsort()
Likewise, the asort() method sorts the values of an associative array in ascending order. Similarly, the arsort() method arranges the values of an associative array in descending order.
<?php
echo 'Original Array...<br>';
$arr1=array('d'=>3, 'j'=>6, 'abc'=>-2, ' p'=>-5, ' xyz'=>2, '@@@'=>7, '12'=>15);
print_r($arr1);
echo '<br>Sorting an associative array in ascending order of values...<br> ';
asort($arr1);
print_r($arr1);
echo '<br>';
echo '<br>Sorting an associative array in descending order of values...<br> ';
arsort($arr1);
print_r($arr1);
?>
Output

ksort() and krsort()
Likewise, the ksort() function sorts the keys of an associative array in ascending order. While the krsort() function sorts the keys in descending function.
<?php
echo 'Original Array...<br>';
$arr1=array('d'=>3, 'j'=>6, 'a'=>-2, 'p'=>-5, 'x'=>2, 'm'=>7, 'e'=>15);
print_r($arr1);
echo '<br>Sorting an associative array in ascending order of keys...<br> ';
ksort($arr1);
print_r($arr1);
echo '<br>';
echo '<br>Sorting an associative array in descending order of keys...<br> ';
krsort($arr1);
print_r($arr1);
?>
Output

array_walk()
Sometimes we need to perform some specific task for each element of an array. In such a case, we need to use a for loop that iterates through each element of the array. Further, we call a function in the loop. However, the array_walk() function simplifies the code. It applies a function to every member of the array. Furthermore, the function that the array_walk() function applies could be an ordinary user-defined function, a built-in function, or an anonymous function.
<?php
function f($k, $v)
{
echo '<tr>';
echo '<td>'.$k.'</td><td>'.$v.'</td>';
echo '</tr>';
}
?>
<?php
echo 'Original Array...<br>';
$arr1=array('d'=>3, 'j'=>6, 'a'=>-2, 'p'=>-5, 'x'=>2, 'm'=>7, 'e'=>15);
print_r($arr1);
echo '<br><br>';
echo '<table border="2">';
echo '<tr><th>Key</th><th>Value</th></tr>';
array_walk($arr1, "f");
echo '</table>';
?>
Output
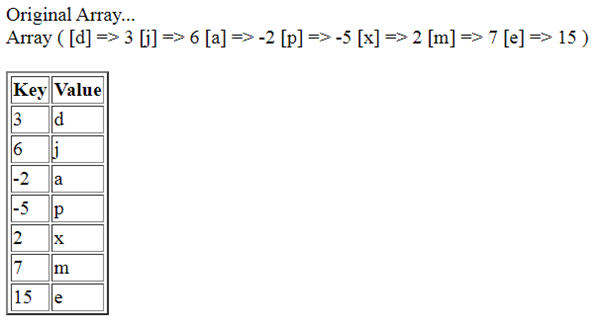
Examples of Built-in Functions of Arrays in PHP – Retrieving Kays and Values
array_keys()
Basically, this function fetches the keys from an associative array.
<?php
echo 'Original Array...<br>';
$arr1=array('d'=>3, 'j'=>6, 'a'=>-2, 'p'=>-5, 'x'=>2, 'm'=>7, 'e'=>15);
print_r($arr1);
echo '<br><br>';
echo 'All Keys of the array....<br>';
$keys=array_keys($arr1);
print_r($keys);
?>
Output
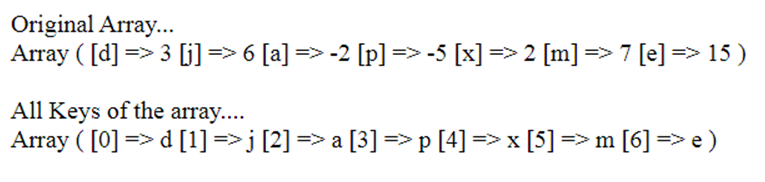
array_values()
In order to retrieve the values in an associative array, we can use the array_values() function.
<?php
echo 'Original Array...<br>';
$arr1=array('d'=>3, 'j'=>6, 'a'=>-2, 'p'=>-5, 'x'=>2, 'm'=>7, 'e'=>15);
print_r($arr1);
echo '<br><br>';
echo 'All Values of the array....<br>';
$values=array_values($arr1);
print_r($values);
?>
Output
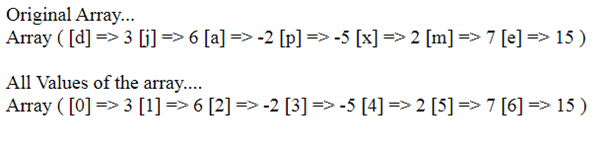