In this article on Learning Simple Queries in SQL, I will explain how to perform simple selection and projection queries on a database table in SQL.
To begin with, let us create a table Course in the Institute database that we have created earlier. The following create command creates the Course table.
create table Course(course_id integer primary key, course_name varchar(10), duration integer, credits integer);
As a result of the execution of the above command, we get the following structure of the Course table.

After that, let us insert certain rows using the following insert commands.
insert into Course(course_id, course_name, duration, credits) values(17, 'BBA', 3, 100)
insert into Course(course_id, course_name, duration, credits) values(20, 'BCA', 3, 100)
insert into Course(course_id, course_name, duration, credits) values(39, 'MBA', 2, 100)
insert into Course(course_id, course_name, duration, credits) values(44, 'MCA', 2, 100)
insert into Course(course_id, course_name, duration, credits) values(324, 'BTECH', 4, 100)
insert into Course(course_id, course_name, duration, credits) values(234, 'LLB', 5, 100)
insert into Course(course_id, course_name, duration, credits) values(28, 'M.Sc.', 2, 100)
The following figure shows the Course table after inserting rows.
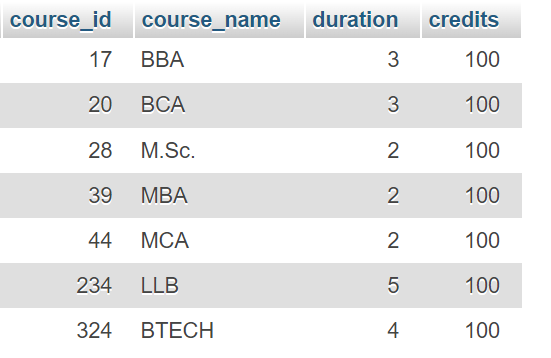
Using Projection and Selection for Learning Simple Queries in SQL
The following section provides the examples of selection and projection queries in SQL.
Projection Queries
Basically, in SQL projection allows us to specify which of the columns we want to retrieve as a result of the query. In other words, the projection query returns the data from chosen columns. The following queries demonstrate the examples of projection.
- Display data from all columns.
SELECT * FROM Course
Output
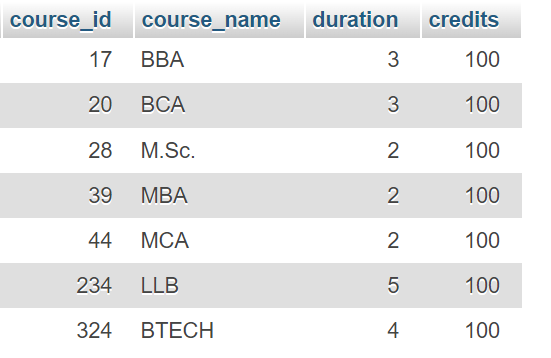
2. Display specific columns
SELECT course_name, duration FROM Course
Output
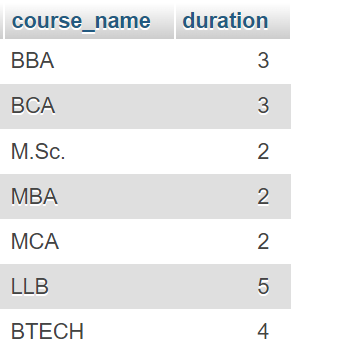
Selection Queries
Likewise, the selection operation allows us to retrieve data from specific rows on the basis of certain condition. In other words, in selection queries we specify the condition in where clause of the select statement. The following queries demonstrate the examples of selection operation.
- Display the courses with duration of more than three years.
SELECT * FROM Course where duration > 3
Output

2. Display courses where the duration falls in the range of three and four years,
SELECT * FROM Course where duration between 3 and 4
Output
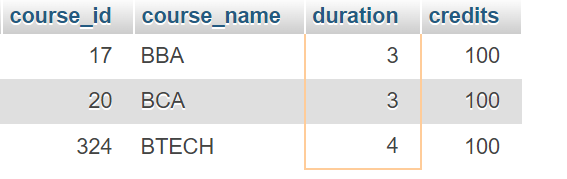
3. Display the courses where duration is either two or three or five years.
SELECT * FROM Course where duration in (2, 3, 5)
Output

4. Display the courses where course name starts with ‘B’ character.
SELECT * FROM Course where course_name LIKE 'B%'
Output

4. Display the courses where course name starts with ‘B’ character and duration is more than three years.
SELECT * FROM Course where course_name LIKE 'B%' and duration > 3
Output

Further Reading
Data Definition Language Commands in SQL
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML