Programmingempire
Microsoft introduced TypeScript in October 2012. So if you are already familiar with web development, then you must have worked with JavaScript and TypeScript is the superset of JavaScript. Why should you consider developing in TypeScript rather than JavaScript which you are already familiar with? In this post on Introduction to Programming in TypeScript, I will discuss several reasons TypeScript can be helpful to web developers.
Before listing the difference between TypeScript and JavaScript, let us first discuss some important points regarding TypeScript
Important Points to Remember about TypeScript
- TypeScript is not like a strictly typed programming language such as C#. The type declaration is not that strict.
- Browsers can’t translate a program in TypeScript. Instead, the TypeScript compiler translates it into JavaScript.
- A TypeScript file has the extension .ts.
- The TypeScript code is transpiled to JavaScript. [Note: A Transpiler converts code in one language to another language]
- Since the TypeScript is a superset of JavaScript, it has support for all JavaScript libraries.
- Unlike JavaScript, TypeScript is an object-oriented language and supports object-oriented features like creating classes, interfaces, and inheritance.
- Finally, the TypeScript language contains most of the features of ES6 (ECMA Script 6).
Difference Between TypeScript and JavaScript
We use both TypeScript and JavaScript in web development and both languages have lots of similarities. However, there are some important differences.
- TypeScript has a compiler and it compiles the code into JavaScript. Whereas, JavaScript is an interpreted language.
- JavaScript is not object-oriented. However, TypeScript possess object-oriented features.
- The compilation of TypeScript code is quite slow as compared to JavaScript.
- TypeScript makes your program error-free by pointing out the compilation errors. Whereas JavaScript lacks this feature.
- There are many important features like static typing and optional parameters that TypeScript supports. However, JavaScript doesn’t support these features.
Introduction to Programming in TypeScript
The programming with TypeScript is quite easy whether you are already familiar with JavaScript or not. After installing TypeScript, you can use any editor like Bracket, Visual Studio Code, or even the Notepad to write a TypeScript program. See the following example of the usage of variables and simple computation. Save the file with name VariablesDemo.ts.
The following program declares three variables of number type and one variable of string type. As shown below, we declare variables using var keyword and specify data type after the : symbol. Then, few arithmetic operations are performed. After that, we use the console.log() method to display the output on the console.
var student_name:string="Jolly"
var marks1:number=78
var marks2:number=57
var marks3:number=86
var total, percentage
total = marks1+marks2+marks3
percentage=(total * 100)/300
var str:string
str="Total Marks: "+total
console.log(str)
str="Percentage: "+percentage
console.log(str)
The above program will compile to a JavaScript file with the name VariablesDemo.js as shown below.
var student_name = "Jolly";
var marks1 = 78;
var marks2 = 57;
var marks3 = 86;
var total, percentage;
total = marks1 + marks2 + marks3;
percentage = (total * 100) / 300;
var str;
str = "Total Marks: " + total;
console.log(str);
str = "Percentage: " + percentage;
console.log(str);
Compile and run the above program. It produces the output as shown below.

- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
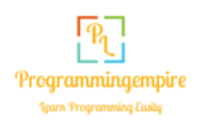