Programmingempire
In this post on Creating Classes in TypeScript, I will explain how we can define a class in TypeScript with the help of a few examples. Also, I will also explain the implementation of inheritance in TypeScript with an example.
Creating Classes in TypeScripteScript allows creating classes and this feature makes it an object-oriented language. However, the class is not as versatile as a class in C#. As a matter of fact, a C# class can contain many different types of class members as compared to a TypeScript class.
A TypeScript class can contain following members:
- fields that represent data members
- constructor that initializes the data members of the class
- function to represent the behavior
Creating Classes in TypeScript
Syntax:
class classname
{
//class members
}
Example 1:
The following example creates a simple class with name A. The only member of the class is a function with the name myfunction which takes a parameter of number type with a default value. Therefore, it possible to call this function without providing an argument as well as with an argument as indicated in the example.
class A
{
myfunction(i:number=10)
{
i++
console.log(`i = ${i}.`)
}
}
var ob=new A()
ob.myfunction(100)
ob.myfunction()
Output:

Example 2:
The following example represents a class of Complex numbers containing two data members real and imag of type number. Also, the class defines a constructor as well as a method to add two complex numbers and a method to display. The constructor initializes the data members of the class with the argument values. However, it is not mandatory to pass parameters when creating an object in this example, since default values are also there.
There are several statements of creating objects and calling specific methods of the class that demonstrate how to work with classes and objects.
Note: It is important to remember that a TypeScript class doesn’t allow multiple constructors.
class Complex
{
real:number
imag:number
constructor(real:number=0, imag:number=0)
{
this.real=real
this.imag=imag
}
add(c1:Complex):Complex
{
var c3 = new Complex()
c3.real=this.real+c1.real
c3.imag=this.imag+c1.imag
return c3
}
show():void
{
console.log(this.real + '+' + this.imag+'i')
}
}
var ob1, ob2, ob3
ob1=new Complex(5, 3)
ob2=new Complex(4, 7)
console.log('First Complex Number')
ob1.show()
console.log('Second Complex Number')
ob2.show()
ob3=ob1.add(ob2)
console.log('Sum')
ob3.show()
Output:

Example 3:
This example demonstrates the implementation of inheritance in TypeScript. To illustrate, class A contains one private data member i and two protected data members j and k which its subclass can inherit. Also, there is a constructor and a compute() method in class A.
Class B inherits class A using the extends keyword and defines its own data members m and n. Also, class B defines its own compute() method. The order of execution of constructors and different methods are shown in the output.
class A
{
private i: number
protected j:number
protected k:number
constructor(i:number=1, j:number=2, k:number=3)
{
this.i=i
this.j=j
this.k=k
console.log("Inside Constructor of Class A")
}
compute():number
{
console.log("Inside Compute method of Class A")
return this.i+this.j+this.k
}
}
class B extends A
{
m:number
n:number
constructor(m:number=10, n:number=20)
{
super()
this.m=m
this.n=n
console.log("Inside Constructor of Class B")
}
compute():number
{
console.log("Inside Compute method of Class B")
return this.j+this.k+this.m+this.n
}
}
var ob1
ob1=new A()
console.log(ob1.compute())
var ob2
ob2=new B()
console.log(ob2.compute())
Output:
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
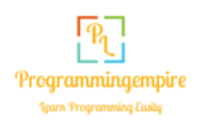