Programmingempire
In this post on Using TypeScript Modules, I will explain the modules in TypeScript with example code. For the purpose of developing a large application, we must keep in mind several aspects of software engineering and code reusability is one of them. Particularly, when some functionality is already developed and available. Basically, modules represent functionality. In essence, modules contain classes, functions, variables, and interfaces.
However, the components of a module are not visible outside the module definition until we export them explicitly.
Example of Creating and Using TypeScript Modules
Now that, we understand the significance of modules, let us create a few. In the following example, we create a class called Person having four properties, a constructor, and four methods. Furthermore, we use the arrow function syntax in creating the methods. It is important to note the use of the export keyword before the class that enables this to be exported. In this way, the class Person becomes available outside the file in which it is defined. Also, the following code is contained in a file called md1.ts.
Exporting a Module
export class Person
{
pid: number;
pname: string;
page: number;
plocation: string;
constructor(p: number, n: string, a:number, l:string)
{
this.pid=p;
this.pname=n;
this.page=a;
this.plocation=l;
}
getPid=()=>this.pid;
getPname=()=>this.pname;
getPage=()=>this.page;
getPlocation=()=>this.plocation;
}
Now, compile the file md1.ts and create another file called md2.ts.

Importing a Module
Importing a Module has following syntax.
import {Name of export entity} from "the path of file containing a export"
In the following code, we import a module which is exported earlier. Therefore, first use import statement to import the class Person. Once, the class is imported we can use it. Hence, we create a subclass Student of the Person and add its own property, constructor, and method. Finally, we create the object of class Student and call its methods.
import {Person} from "./md1"
class Student extends Person
{
course: string;
constructor(p:number, n: string, a: number, l:string, c:string)
{
super(p,n,a,l);
this.course=c;
}
getCourse=()=>this.course;
}
var ob=new Student(1, "Anu", 12, "Delhi", "MCA");
console.log("Person ID: "+ob.getPid());
console.log("Person Name: "+ob.getPname());
console.log("Person Age: "+ob.getPage());
console.log("Location: "+ob.getPlocation());
console.log("Course of Student: "+ob.getCourse());
Output

Importing Several Members of a Module
In a similar way, we can import several members of a module. Let us consider another example in which we export a constant and an interface.
Exporting a Constant and an Interface
Basically, we create a file md3.ts and declare a constant and define an interface in it. Further, we export keywords for both of the members – the constant pi and the interface area. Now compile this file.
export const pi = 3.14
export interface area
{
radius: number;
findarea():number;
}
Importing the Members of a Module
Now that, we already have a module available with us. Therefore, create another file called md4.ts and write the import statement as given below. After that, we create a class called Circle that implements the interface area. Also, the class Circle implements the method findarea() that makes use of the imported constant pi.
import * as r from "./md3"
class Circle implements r.area
{
radius: number;
constructor(rd: number)
{
this.radius=rd;
}
findarea=()=>r.pi*this.radius*this.radius;
}
let ob=new Circle(4.1);
console.log("Radius of Circle: "+ob.radius);
console.log("Area of Circle: "+ob.findarea());
Output

Summary
In this article on Using Modules in TypeScript, creating and using modules in TypeScript are explained. As I said earlier, modules support reusability, which means you can create functionality in the form of modules and reuse it again by using the import statement. Indeed, we can export any construct like interface, class, or variable and later use it in any other TypeScript file using the import statement.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
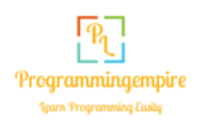