In this article on Exploring Data Structures in Java, I will give an overview of some of the commonly used data structures in Java.
Overview of Common Data Structures in Java
- Arrays. Arrays are the most basic and simple data structure. We use arrays to store a fixed number of homogeneous elements. Further, all elements have the same data type. Also, they reside in contiguous memory locations.
- ArrayList. Similarly, an ArrayList is a dynamic array implementation that can grow and shrink in size as elements are added or removed. Moreover, it provides several methods to manipulate elements. For instance, an ArrayList has methods for adding, removing, and searching for elements.
- LinkedList. Likewise, it is a linear data structure that consists of nodes connected through pointers. Each node contains a data element and a reference to the next one. In fact, LinkedLists provide efficient insertion and deletion operations. However, they are slower than ArrayLists when it comes to random access.
- Stack. It is a LIFO data structure and permits elements to be added and removed from one end only. Basically, the two main operations on a Stack are push() to add an element and pop() to remove an element from the top.
- Queue. Similarly, it is a FIFO data structure that allows elements to be added at one end and removed from the other end. Like Stack, the two main operations on a Queue are enqueue() to add an element and dequeue() to remove the front element.
- HashSet. In general, a HashSet is a collection that contains no duplicate elements. It uses a hash table to store the elements. Furthermore, it provides constant-time performance for operations like adding, removing, and searching for elements.
- HashMap. Similarly, a HashMap is a collection that stores key-value pairs. Also, it has constant-time performance for operations like adding, removing, and searching for elements based on the key.
Summary
To summarize, these are just a few examples of the data structures available in Java. So, depending on the requirements of your application, you can choose the appropriate one. Moreover, there are several other data structures that you can use such as Trees, Graphs, and Heaps.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
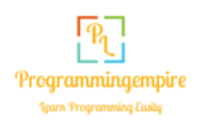