Programmingempire
This article describes Working with Classes and Objects in Java. Basically, class is a user-defined type. While an object is a real-world entity. Further, an object has attributes and also has a behavior. Since Java is an object-oriented language, a Java program comprises one or more classes containing certain members.
Syntax of Creating a Class in Java
<access-modifier> class <class-name>{
//class members
}
//Example
public class MyClass{
int a, b;
public MyClass(int a, int b)
{
this.a=a;this.b=b;
}
public void show()
{
System.out.println("a = "+a+" b = "+b);
}
}
So, we declare a class using the keyword class. Also, the access modifier is public. Furthermore, if a class is declared as public then it must be saved in a file with the same name as the public class. Hence, we name our Java file MyClass.java.
Creating Objects
Likewise, we need to create objects also to use the functionality provided by the class. In Java, we create objects using the new keyword. For example, look at the following code for instantiating MyClass.
MyClass ob=new MyClass(67, 89);
ob.show();
The following output will be produced by the above Code.
a=67 b=89
Members of a Java Class
In general, a Java class can have instance variables, methods, constructors, constants, instance and static blocks, and inner classes as its members. The following example demonstrates the members of a class in Java.
File Name: Main.java
public class Main
{
public static void main(String[] args) {
Result ob=new Result(57);
ob.show();
}
}
class Result{
static final int credits=4;
String courseName;
int courseDuration;
int total_marks;
{
courseName="BCA";
courseDuration=3;
}
public Result(int total_marks)
{
this.total_marks=total_marks;
}
public void show()
{
System.out.println("Course Name: "+courseName);
System.out.println("Course Duration: "+courseDuration);
System.out.println("Course Total: "+total_marks*credits);
}
}
Important Points Regarding the above Program
As can be seen, the class Result contains a constant called credits which is declared as static and final. Also, it has three instance variables with names courseName, courseDuration, and total_marks. Further, the class Result contains an instance block in order to initialize two of the instance variables, that is, the courseName, and courseDuration. the third instance variable total_marks is initialized by the constructor. Moreover, the class has an instance method with the name show(). Once, the class definition is complete we can create its objects and call its methods. So, we instantiate the class in the main() method that the class Main contains.
Output:

- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
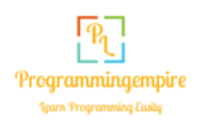