Programmingempire
Basically, Standard Template Library (STL) in C++ is a library of data structures. Hence, by using STL, we need not bother about implementing the commonly used data structures. Instead, we can focus on application-specific tasks. Therefore, Standard Template Library in C++ supports the reusability of the code.
Another benefit that we get by using STL is that our code becomes more robust and efficient. Even it is possible to extend STL by adding algorithms.
Components of Standard Template Library in C++
The following list describes the major components found in STL.
- Basically, Algorithms describe the operations that can be performed on the stored data. While, there are large number of algorithms available in STL, the most commonly used ones are searching and sorting.
- While algorithms specify operations on data, the Containers store the data. In fact, the Containers represent commonly used data structures such as vector, list, and arrays.
- Another component of STL is Iterators. In order to process a sequence of values, we can use iterators.
- In fact, Functions are the objects that represent the overloading of function call operator (). The Function objcts are also known as Functors.
Header Files for using Standard Template Library
Although STL contain a large number of header files, some examples are <algorithm>, <array>, <stack>, <iterator>, <list>, <vector>, <functional>, <set>, <map>, and so on.
A Basic Example of Using Standard Template Library in C++
The following code shows a basic example of using STL.
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
int myarray[] = {78, 12, 90, -34, 29};
cout << "Before Sorting : \n";
for(int i=0;i<5;i++)
cout<<myarray[i]<<" ";
sort(myarray, myarray+5);
cout << "\n\n After Sorting : \n";
for(int i=0;i<5;i++)
cout<<myarray[i]<<" ";
return 0;
}
Output

As shown in the code, the sort() function is available in <algorithm> header file. In fact, the sort() function accepts three parameters – first, last which are of the type RandomAccessIterator, and a comparison predicate, which is optional. While, here we use myarray as the first position, and myarray+5 asthe last position. Also, the comparison predicate represents ascending order by default. In order to perform sorting in descending order, we can use std::greater<int>() function. The following code shows an example of soring in descending order.
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
int myarray[] = {78, 12, 90, -34, 29};
cout << "Before Sorting : \n";
for(int i=0;i<5;i++)
cout<<myarray[i]<<" ";
sort(myarray, myarray+5, std::greater<int>());
cout << "\n\n After Sorting : \n";
for(int i=0;i<5;i++)
cout<<myarray[i]<<" ";
return 0;
}
Output

- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
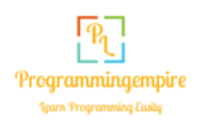