The following list presents a C++ Practice Exercise.
Solve the Following C++ Practice Exercise
- Write a program to implement the ‘Inline function’
- Implement call by reference and return by reference using class in a C++ program. [Hint. Assume necessary functions]
- Write a program to implement the friend function by taking some real-life examples
- Implement ‘Function Overloading’ in C++.
- Write a program to implement Parameterized Constructor, Copy Constructor, and Destructor
- When should we use a constructor? Write a program to show the usage of constructor in base and derived classes, in multiple inheritance
- When should we use containership? Create a program in C++ to show the implementation of ‘containership’
- Write a program to show swapping using template function (Generic)
- When do we need to use exception handling? Write a program to implement ‘Exception Handling’
- Create an application in C++ to read and write values through objects using file handling
- Create a class employee which has the name, age, and address of the employee, including functions getdata() and showdata(), getdata() takes the input from the user, showdata() display the data in the following format: Name: Age: Address: in separate lines.
Application Based C++ Practice Exercise
- Write a C++ program to create a class called MyAccount with acc_no and acc_bal as two data members. While acc_no is integer, the data member acc_bal is of floating-point type. Also, define three member functions in the class including a constructor: (i) The constructor sets the values of data members using parameters and some initial values. So, define both default as well as parameterized constructors. (ii) In order to perform input transactions, create a single function called performTransaction() that reads a character value for the type of transaction(‘D’ for deposit and ‘W’ for withdrawal), as well as the amount. As a result it should update the balance. Also, it should return its value. (iii) Finally, define a function called display(), to show on the screen the account number, and the balance.
- Write a C++ program to create a class called CAccount that contains two private data elements, an integer accountNumber, and a floating-point accountBalance, and three member functions: (i) A constructor that allows the user to set initial values for accountNumber and accountBalance and a default constructor that prompts for the input of the values for the above data numbers. (ii) A function called inputTransaction, which reads a character value for transactionType(‘D’ for deposit and ‘W’ for withdrawal), and a floating point value for transactionAmount, which updates accountBalance. (iii) A function called printBalance, which prints on the screen the accountNumber and accountBalance.
- Define a class Counter which contains an int variable count defined as static and a static function Display () to display the value of count. Whenever an object of this class is created count is incremented by 1. Use this class in the main() method to create multiple objects of this class and display the value of count each time
- Write a program to add and subtract two complex numbers using classes
- Create a program to overload Binary + to add two similar types of objects. (Both with and without using friend functions)
- Write a program to implement += and = operator
- When operator overloading should be done? Develop a program in C++ to convert meter to centimeter and vice versa, using data conversions and operator overloading
- When to use streams in C++? Display the count of digits, alphabets, and spaces, stored in a text file, using streams in a C++ program.
- Implement the class hierarchy of fig. 1 considering appropriate data members and member functions
- Create a C++ program to implement the class hierarchy of fig. 2 considering appropriate data members and member functions (use Virtual functions).


Standard Template Library in C++
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
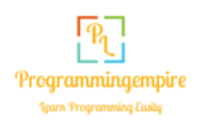