Programmingempire
This article shows programs to Find Armstrong Number in Java. Basically, checking for an Armstrong is an important programming problem to learn a programming language. Also, it helps in developing programming logic. Meanwhile, you can find more information on Armstrong numbers here. In order to write a program, first, we need to develop its logic.
Therefore, we can write an algorithm for this problem as given below.
begin
low <- read_lower_limit_of_range
high <- read_higher_limit_of_range
result <- 0
n <- low
while(n<=high)
begin
len <- find_number_of_digits(n)
n1 <- n
while(n1<>0)
begin
remainder <- n1 MOD 10
result <- result + remainder ^len
n1 <- n1/10
end
if n1=result
print(n1)
end if
result <- 0
end
Now that, we have developed the logic, we can implement it.
Program to Find Armstrong Number in Java
The following program finds and prints all Armstrong numbers in the specified range. To begin with, first, we need to read the lower bound and upper bound of the range in respective variables. Further, we declare necessary variables. Accordingly, we use variables for storing result, remainder, and the number that we currently checking.
In order to work on all numbers within the range, we use a for loop. Further, in the loop, first we compute the number of digits in the current number. After that, within another loop we need to perform following steps. Also, before starting the inner loop we must set the variable result to zero.
- At first, we find remainder of the number
- After that, we add remainder raised to the power number of digits to the result
- Finally, divide the number by 10
The above inner loop executes till the number becomes zero. Therefore now we can check whether the number that we are currently checking equals to the result computed within the inner loop. Accordingly, we can display the number. Lastly, we can set the result variable to zero again inorder to check for the next number in the range.
import java.io.*;
public class Main
{
public static void main(String[] args) throws IOException {
System.out.println("Checking Armstrong Number in Given Range...");
System.out.println("Enter Starting Value of the Range: ");
BufferedReader bfrd=new BufferedReader(new InputStreamReader(System.in));
int low=Integer.parseInt(bfrd.readLine());
System.out.println("Enter Last Value of the Range: ");
int high=Integer.parseInt(bfrd.readLine());
int n1;
int remainder;
int result = 0;
for(int n=low;n<=high;n++)
{
String str = Integer.toString(n);
int strlen = str.length();
n1 = n;
while(n1!=0)
{
remainder = n1 % 10;
result += (int)Math.pow(remainder, strlen);
n1 = n1 / 10;
}
if(n==result)
{
System.out.print(n + " ");
}
result = 0;
}
}
}
Output
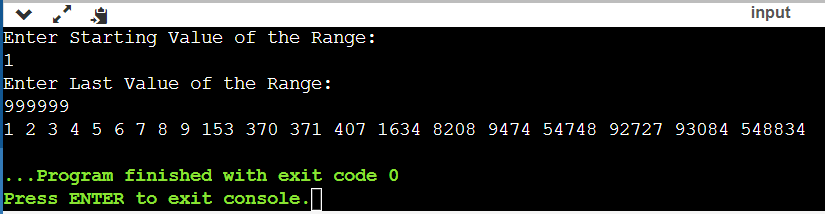
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
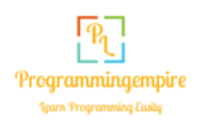