In this article on Examples of Global Variables in PHP, I will explain how to create and use global variables.
Basically, there are 9 superglobals in PHP which remain available throughout the scripts. In other words, these superglobals can be accessed in any part of the script. These superglobals include $GLOBALS, $_SERVER, $_POST, $_GET, $_REQUEST, $_FILES, $_ENV, $_COOKIE, and $_SESSION.
Understanding $GLOBALS
As can be seen, $GLOBALS is a superglobal variable that we can use to access the global variables. Meanwhile, the global variables referred to with the help of $GLOBALS remain available in any part of the PHP script. Therefore, we can access these global variables within any function or outside it.
The following section presents some Examples of Global Variables in PHP
The following code shows how to use global variables to swap two numbers. At first, create two variables x and y, and initialize them with values. After that, we define a function swap() that doesn’t take any parameter. Because the variables x and y are used as global variables in the function. Therefore, the change in their values is visible outside the function after we call the function swap().
<?php
$x=10;
$y=20;
echo 'Before Swapping...<br>';
echo 'x = ',$x,'<br>y = ',$y,'<br>';
function swap()
{
$t=$GLOBALS['x'];
$GLOBALS['x']=$GLOBALS['y'];
$GLOBALS['y']=$t;
}
swap();
echo 'After Swapping...<br>';
echo 'x = ',$x,'<br>y = ',$y,'<br>';
?>
Output
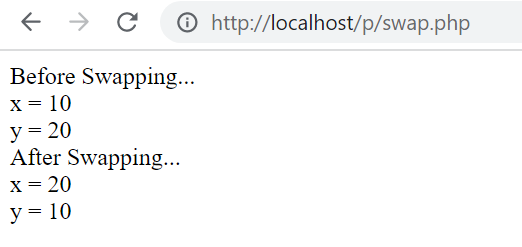
The following code shows another example of the global variables. Within the function, we create the variable x as a local variable and a global variable. Therefore within the function, $x represents the local variable, whereas, $GLOBALS[‘X’] represents a global variable. When we retrieve the value of $GLOBALS[‘x’], we get the updated value outside the function.
<?php
function f1()
{
$x=100;
$GLOBALS['x']=200;
echo 'Local variable x = ',$x;
echo '<br>Global variable x = ',$GLOBALS['x'];
}
f1();
echo '<br>variable x outside the function: ',$GLOBALS['x'];
?>
Outside
