In this article, I will explain What is Kruskal’s Algorithm and How Does it Work.
Kruskal’s algorithm is a greedy algorithm used to find the minimum spanning tree of a weighted, undirected graph. It works by initially sorting all the edges in the graph by their weights, and then adding edges to the minimum spanning tree in increasing order of weight, as long as the addition of the edge does not create a cycle in the tree.
At each step, Kruskal’s algorithm considers the next edge in the sorted list and checks if it connects two disjoint sets of vertices in the tree. If it does, the edge is added to the tree, and the two sets are merged. Otherwise, the algorithm discards the edge, and the algorithm moves on to the next edge. This process is repeated until all vertices are included in the minimum spanning tree or all edges have been considered.
The time complexity of Kruskal’s algorithm is O(E log E), where E is the number of edges in the graph. This makes it an efficient algorithm for finding the minimum spanning tree of large graphs.
When to Use Kruskal’s Algorithm?
- Sparse Graphs. In fact, Kruskal’s algorithm works well for sparse graphs, where the number of edges is significantly smaller than the maximum possible number of edges. Actually, it’s efficient for such graphs because it doesn’t involve a lot of unnecessary edge comparisons.
- Edge-Weighted Graphs. Kruskal’s algorithm is designed for graphs where each edge has a weight or cost associated with it. It finds the MST that minimizes the total weight of the edges, making it suitable for various applications like network design or cost optimization.
- Disconnected Graphs. Likewise, if you have a disconnected graph (a graph with multiple connected components), Kruskal’s algorithm can be applied to each connected component separately to find the minimum spanning tree for each component.
- Edge Weight Variability. Similarly, Kruskal’s algorithm can handle graphs with varying edge weights, including graphs with both positive and negative weights. However, it cannot handle graphs with negative weight cycles.
- Parallel Processing. Also, Kruskal’s algorithm is inherently parallelizable, which makes it suitable for implementation in parallel or distributed computing environments.
- Greedy Approach. Kruskal’s algorithm is based on a greedy approach, meaning it makes locally optimal choices at each step. This makes it easy to understand and implement.
- Alternative to Prim’s Algorithm. Kruskal’s algorithm is an alternative to another popular MST algorithm called Prim’s algorithm. While both algorithms aim to find the minimum spanning tree, we prefer Kruskal’s algorithm in situations where the graph is dense or where you want to emphasize edge weights rather than vertex-based considerations.
- Efficiency. Kruskal’s algorithm has a time complexity of O(E log E), where E is the number of edges in the graph. This makes it efficient for many practical scenarios, especially when the graph is not too large.
However, there are situations where Kruskal’s algorithm may not be the best choice.
When Not to Use Kruskal’s Algorithm?
- Graphs with Negative Weight Cycles. Kruskal’s algorithm cannot handle graphs that contain negative weight cycles because it may keep adding negative-weight edges to the MST indefinitely.
- Connected Components Required. Also, Kruskal’s algorithm assumes that the input graph is connected. Therefore, if you have a disconnected graph and need to find a minimum spanning forest (a set of MSTs for each connected component), you would need to modify the algorithm or apply it separately to each component.
To summarize, Kruskal’s algorithm is a valuable tool for finding minimum spanning trees in connected, undirected graphs, especially in scenarios involving sparse graphs with edge weights. Moreover, it is versatile, efficient, and relatively easy to implement. However, it’s essential to consider the specific characteristics of your problem and graph before choosing an algorithm for MST computation.
Further Reading
Top 30 Algorithms You Must Know for Coding Interview
What is Prim’s Algorithm and how Does it Work?
What is Huffman Coding Algorithm?
How to Create Hashing Table Using Open Addressing?
How to Perform Perfect Hashing?
Innovative Project Ideas in Terraform
How to Perform Universal Hashing?
What is Randomized Select Algorithm?
When should we prefer to React over PHP?
What is Rabin-Karp Algorithm and How Does it Work?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
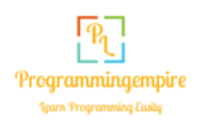