Programmingempire
This article contains some more JSTL Examples. To begin with, the three tags that provide the functionality of the switch…case statement in Java are discussed.
c:choose Tag
Basically this tag work like the switch…case statement in Java and provides a mutually exclusive conditional operations.
c:when Tag
Likewise, the c:when tag works as a Case statement in switch….case statement of Java. Therefore, witin the c:choose tag, we can specify several c:when tags according to the conditions we want to check.
c:otherwise Tag
Similarly, c:otherwise works like default statement in switch..case. So, we provide it to specify the set of statements when all the conditions specified in c:when tags don’t match. The following code shows an example of c:choose, c:when, and c:otherwise tags.
JSTL Examples of c:choose, c:when, and c:otherwise
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>c:choose Example</title>
</head>
<body>
<c:set var="choice" value="78"/>
<c:choose>
<c:when test = "${choice>90}">
<c:out value="A+"/>
</c:when>
<c:when test = "${choice>80}">
<c:out value="A"/>
</c:when>
<c:when test = "${choice>70}">
<c:out value="B+"/>
</c:when>
<c:when test = "${choice>65}">
<c:out value="B"/>
</c:when>
<c:when test = "${choice>55}">
<c:out value="C"/>
</c:when>
<c:when test = "${choice>40}">
<c:out value="D"/>
</c:when>
<c:otherwise>
<c:out value="F"></c:out>
</c:otherwise>
</c:choose>
</body>
</html>
Output
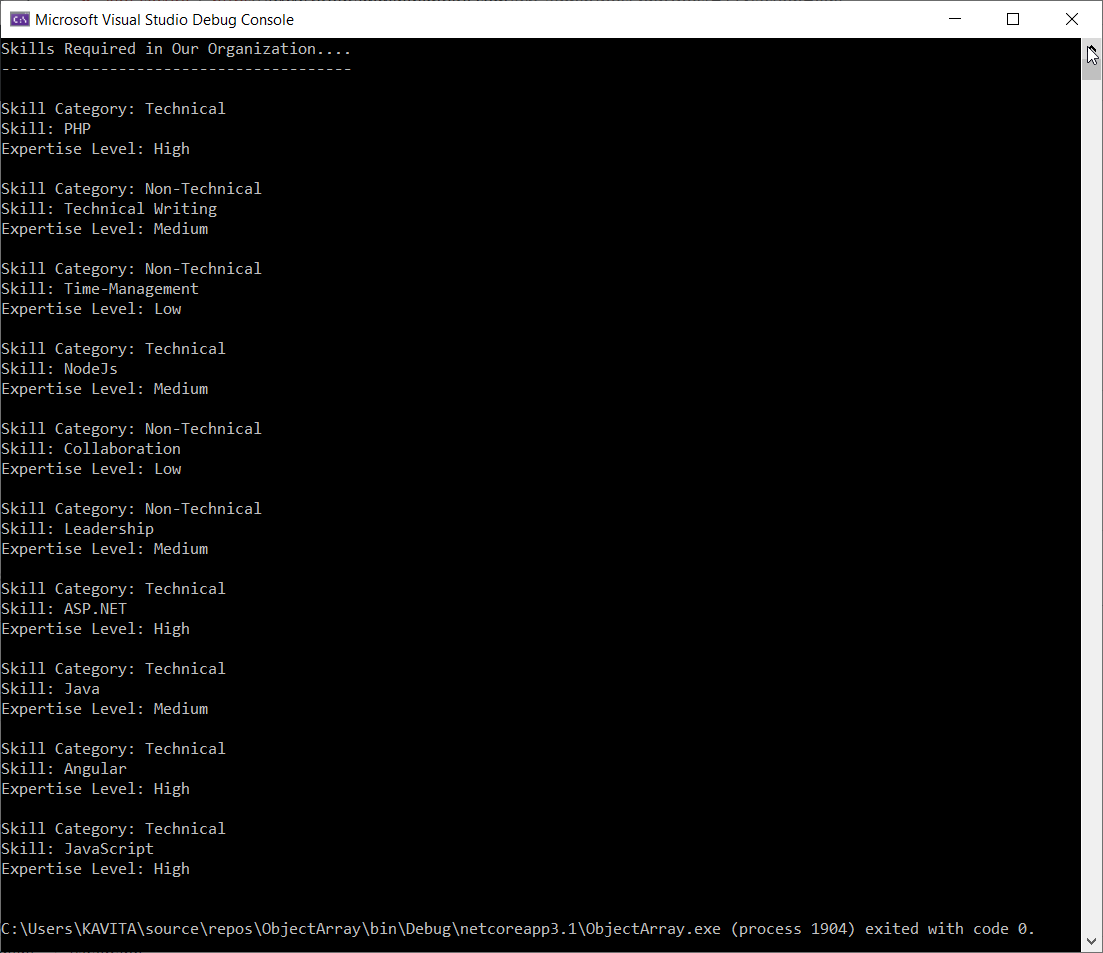
c:forTokens Tag
Meanwhile, suppose we have a string and we want to break it into tokens, then we can use the c:forTokens tag. Basically, this tag iterates through all the tokens which are separated by specified delimiters in a string. the following code shows an example of this tag.
JSTL Examples of c:forTokens
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>c:forTokens Example</title>
</head>
<body>
<c:forTokens items="ab;bc;cd;de;ef;fg;gh" delims=";" var="i">
<c:out value="${i}"/>
<br/>
</c:forTokens>
</body>
</html>
Output
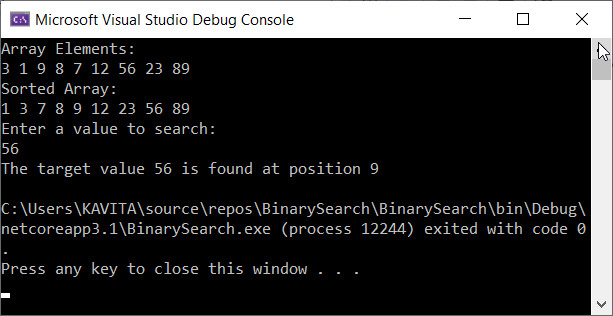
c:catch Tag
Basically, the c:catch tag handles the run-time exceptions and gracefully indicates the description of errors. In other words, this tag catches any exception that occurs in the body. The following code shows an example of c:catch tag.
JSTL Examples of c:catch
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>c:catch Example</title>
</head>
<body>
<c:catch var="cep">
<%
int x=78/0;
%>
</c:catch>
<c:if test="${cep!=null}">
<c:out value="Exception: ${cep}"/>
<br/>
<c:out value="${cep.message}"/>
</c:if>
</body>
</html>
Output
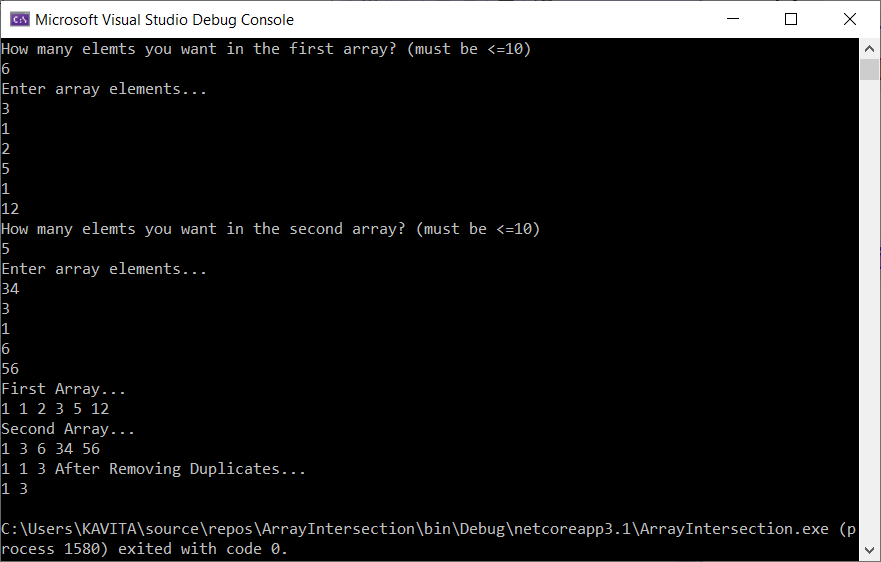
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
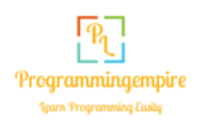