Programmingempire
In this post on How to Create Accessor Decorators in TypeScript, I will explain the accessor decorators with few examples. Analogous to other decorators in TypeScript, the accessor decorators also represent the metadata. However, they are used with the get and set accessors of the properties of a class.
Certainly, the accessor decorators are placed just before a get or a set accessor of a property. Moreover, these decorators can also alter the definition of a particular accessor.
Before going into detail about accessor decorators, let us first create a class that contains properties with get and set accessors as shown below. After that, compile and run the program. However, you need to target ES5 for compiling the code that uses accessors.
class Car
{
private _ModelName: string;
private _year: number;
public get ModelName(){ return this._ModelName;}
public set ModelName(s:string){this._ModelName=s;}
public get year(){return this._year;}
public set year(y: number){this._year=y;}
}
let c1=new Car();
c1.ModelName="Creta";
c1.year=2019;
let c2=new Car();
c2.ModelName="Compass";
c2.year=2020;
console.log("First Object: ");
console.log(`Model name: ${c1.ModelName}, Year: ${c1.year}`);
console.log();
console.log("Second Object: ");
console.log(`Model name: ${c2.ModelName}, Year: ${c2.year}`);
console.log();
Output

Now that, we have properties with accessors in our class, let us create the accessor decorators. As an illustration, we name the decorator as MyDecorator and apply it to the accessors of the property ModelName. Further, it is important to note that you can apply the decorator to only one of the get or set accessors.
function MyDecorator(
target: Object,
propertyKey: string,
descriptor: TypedPropertyDescriptor<string>
) {
console.log(descriptor);
}
class Car
{
private _ModelName: string;
private _year: number;
@MyDecorator
public get ModelName(){ return this._ModelName;}
public set ModelName(s:string){this._ModelName=s;}
public get year(){return this._year;}
public set year(y: number){this._year=y;}
}
let c1=new Car();
c1.ModelName="Creta";
c1.year=2019;
console.log("First Object: ");
console.log(c1.ModelName);
console.log(`Year: ${c1.year}`);
console.log();
Output

As can be seen in the output, the descriptor in the accessor decorator has four components – get, set, enumerable, and configurable. Although, get, and set are common to use, but the other two are worth mentioning.
Basically, enumerable indicates that the data can be displayed in for..in loop. However, configurable means that the data can be modified. Finally, let us look at another example consisting of a decorator that manipulates the set accessor.
function MyDecorator(
target: Object,
propertyKey: string,
descriptor: TypedPropertyDescriptor<string>
) {
let a=descriptor.set;
console.log(a);
let s = descriptor.set;
descriptor.set = function (value: any) {
console.log("Manipulating Set Accessor!");
return s.call(this, { ...value })
}
}
class Car
{
private _ModelName: string;
private _year: number;
@MyDecorator
public get ModelName(){ return this._ModelName;}
public set ModelName(s:string){this._ModelName=s;}
public get year(){return this._year;}
public set year(y: number){this._year=y;}
}
let c1=new Car();
c1.ModelName="Creta";
c1.year=2019;
console.log("First Object: ");
console.log(c1.ModelName);
console.log(`Year: ${c1.year}`);
console.log();
Output

Summary
To sum up, in this article on How to Create Accessor Decorators in TypeScript, I have explained accessor decorators using some examples. Besides, working on examples, the important points related to accessor decorators are also discussed such as the components of descriptors and altering an accessor.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
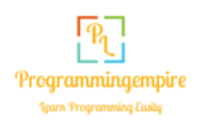